Python: Convert a given dictionary to a list of tuples
77. Transform Dictionary into List of Tuples
Write a Python program to transform a dictionary into a list of tuples.
- Use dict.items() and list() to get a list of tuples from the given dictionary.
Sample Solution:
Python Code:
# Define a function 'test' that takes a dictionary 'd' and converts it into a list of tuples using the 'items' method.
def test(d):
# Use the 'items' method to convert the dictionary into a list of key-value pairs (tuples).
return list(d.items())
# Create a dictionary 'd' with key-value pairs.
d = {'Red': 1, 'Green': 3, 'White': 5, 'Black': 2, 'Pink': 4}
# Print the original dictionary.
print("Original Dictionary:")
print(d)
# Call the 'test' function to convert the dictionary 'd' into a list of tuples (key-value pairs).
print("\nConvert the said dictionary to a list of tuples:")
print(test(d))
Sample Output:
Original Dictionary: {'Red': 1, 'Green': 3, 'White': 5, 'Black': 2, 'Pink': 4} Convert the said dictionary to a list of tuples: [('Red', 1), ('Green', 3), ('White', 5), ('Black', 2), ('Pink', 4)]
Flowchart:
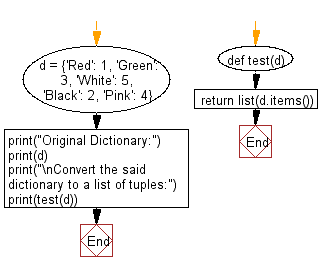
For more Practice: Solve these Related Problems:
- Write a Python program to convert a dictionary into a list of (key, value) tuples using the items() method.
- Write a Python program to use list comprehension to generate a list of tuples from a dictionary.
- Write a Python program to sort a dictionary's items and return them as a list of tuples.
- Write a Python program to iterate over a dictionary and append each key-value pair as a tuple to a new list.
Python Code Editor:
Previous: Write a Python program to combine two lists into a dictionary, where the elements of the first one serve as the keys and the elements of the second one serve as the values. The values of the first list need to be unique and hashable.
Next: Write a Python program to create a flat list of all the keys in a flat dictionary.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics