Python: Convert a list of dictionaries into a list of values corresponding to the specified key
73. Extract List of Values from List of Dictionaries
Write a Python program to convert a list of dictionaries into a list of values corresponding to the specified key.
- Use a list comprehension and dict.get() to get the value of key for each dictionary in lst.
Sample Solution:
Python Code:
Sample Output:
Original list of dictionaries: [{'name': 'Theodore', 'age': 18}, {'name': 'Mathew', 'age': 22}, {'name': 'Roxanne', 'age': 20}, {'name': 'David', 'age': 18}] Convert a list of dictionaries into a list of values corresponding to the specified key: [18, 22, 20, 18]
Flowchart:
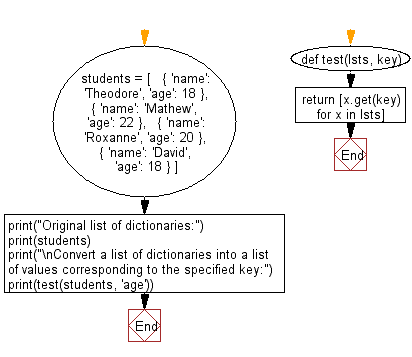
For more Practice: Solve these Related Problems:
- Write a Python program to extract values corresponding to a specified key from a list of dictionaries using list comprehension.
- Write a Python program to iterate over a list of dictionaries and collect the values for a given key, handling missing keys gracefully.
- Write a Python program to use the map() function to retrieve values from each dictionary for a specified key.
- Write a Python program to implement a function that returns a list of values for a given key from a list of dictionaries.
Python Code Editor:
Previous: Write a Python program to invert a dictionary with unique hashable values.
Next: Write a Python program to create a dictionary with the same keys as the given dictionary and values generated by running the given function for each value.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics