Python: Retrieve the value of the nested key indicated by the given selector list from a dictionary or list
Write a Python program to retrieve the value of the nested key indicated by the given selector list from a dictionary or list.
Sample Solution:
Python Code:
# Import the 'reduce' function from the 'functools' module and the 'getitem' function from the 'operator' module.
from functools import reduce
from operator import getitem
# Define a function 'test' that takes a dictionary 'd' and a list of 'selectors'.
def test(d, selectors):
# Use 'reduce' to successively apply 'getitem' with each element in 'selectors' on the dictionary 'd'.
# This effectively drills down into the dictionary using the sequence of selectors.
return reduce(getitem, selectors, d)
# Create a nested dictionary 'users'.
users = {
'Carla ': {
'name': {
'first': 'Carla ',
'last': 'Russell'
},
'postIds': [1, 2, 3, 4, 5]
}
}
# Call the 'test' function with two sets of selectors to access specific values within the 'users' dictionary.
# The first call accesses Carla's last name, and the second call accesses one of Carla's post IDs.
print(test(users, ['Carla ', 'name', 'last']))
print(test(users, ['Carla ', 'postIds', 1]))
Sample Output:
Russell 2
Flowchart:
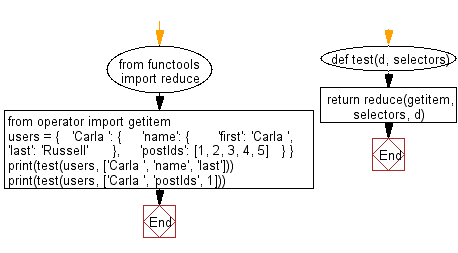
Python Code Editor:
Previous: Write a Python program to map the values of a given list to a dictionary using a function, where the key-value pairs consist of the original value as the key and the result of the function as the value.
Next: Write a Python program to invert a dictionary with unique hashable values.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics