Python: Map dictionary
70. Map List Values to Dictionary Using a Function
Write a Python program to map the values of a given list to a dictionary using a function, where the key-value pairs consist of the original value as the key and the result of the function as the value.
- Use map() to apply fn to each value of the list.
- Use zip() to pair original values to the values produced by fn.
- Use dict() to return an appropriate dictionary.
Sample Solution:
Python Code:
# Define a function 'test' that takes two arguments: 'itr' (an iterable) and 'fn' (a function).
def test(itr, fn):
# Use the 'zip' function to pair each element from 'itr' with the result of applying 'fn' to that element.
# Convert the zipped pairs into a dictionary where the elements from 'itr' are keys, and the results of 'fn' are values.
return dict(zip(itr, map(fn, itr)))
# Call the 'test' function with an iterable [1, 2, 3, 4] and a lambda function that squares its input.
# The lambda function calculates the square of each element in the iterable.
result = test([1, 2, 3, 4], lambda x: x * x)
# Print the resulting dictionary.
print(result)
Sample Output:
{1: 1, 2: 4, 3: 9, 4: 16}
Flowchart:
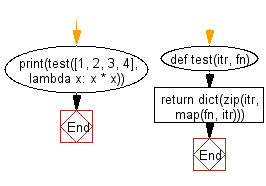
For more Practice: Solve these Related Problems:
- Write a Python program to convert a list of numbers into a dictionary where each key is the number and its value is the square of the number.
- Write a Python program to map a list of strings to a dictionary where each key is the string and the value is its length.
- Write a Python program to implement a function that takes a list and a function, returning a dictionary mapping each element to the function’s result.
- Write a Python program to use dictionary comprehension to map each element of a list to its factorial value.
Python Code Editor:
Previous: Write a Python program to group the elements of a given list based on the given function.
Next: Write a Python program to retrieve the value of the nested key indicated by the given selector list from a dictionary or list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics