Python: Groups the elements of a given list based on the given function
69. Group Elements of a List Based on a Function
Write a Python program to group the elements of a given list based on the given function.
- Use collections.defaultdict to initialize a dictionary.
- Use fn in combination with a for loop and dict.append() to populate the dictionary.
- Use dict() to convert it to a regular dictionary.
Sample Solution:
Python Code:
# Import the 'defaultdict' class from the 'collections' module.
from collections import defaultdict
# Import the 'floor' function from the 'math' module.
from math import floor
# Define a function 'test' that takes a list 'lst' and a function 'fn' as arguments.
def test(lst, fn):
# Create a 'defaultdict' object 'd' with a default value of an empty list.
d = defaultdict(list)
# Iterate through the elements 'el' in the input list 'lst'.
for el in lst:
# Apply the function 'fn' to the current element 'el' to determine the group key.
key = fn(el)
# Append the current element 'el' to the list associated with the calculated key.
d[key].append(el)
# Convert the 'defaultdict' 'd' back to a regular dictionary and return it.
return dict(d)
# Define a list of numbers 'nums' and a function 'floor' for rounding down.
nums = [7, 23, 3.2, 3.3, 8.4]
# Print the original list and specify the function used for grouping.
print("Original list & function:")
print(nums, " Function name: floor:")
# Call the 'test' function with 'nums' and the 'floor' function as arguments,
# and print the result of grouping based on the 'floor' function.
print("Group the elements of the said list based on the given function:")
print(test(nums, floor))
print("\n")
# Define a list of colors 'colors'.
colors = ['Red', 'Green', 'Black', 'White', 'Pink']
# Print the original list and specify the function used for grouping.
print("Original list & function:")
print(colors, " Function name: len:")
# Call the 'test' function with 'colors' and the 'len' function as arguments,
# and print the result of grouping based on the 'len' function.
print("Group the elements of the said list based on the given function:")
print(test(colors, len))
Sample Output:
Original list & function: [7, 23, 3.2, 3.3, 8.4] Function name: floor: Group the elements of the said list based on the given function: {7: [7], 23: [23], 3: [3.2, 3.3], 8: [8.4]} Original list & function: ['Red', 'Green', 'Black', 'White', 'Pink'] Function name: len: Group the elements of the said list based on the given function: {3: ['Red'], 5: ['Green', 'Black', 'White'], 4: ['Pink']}
Flowchart:
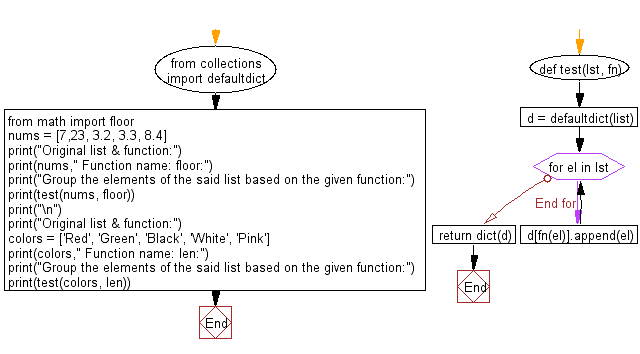
For more Practice: Solve these Related Problems:
- Write a Python program to group elements of a list by applying the floor function and output a dictionary of groups.
- Write a Python program to group words from a list by their length using a lambda function and dictionary comprehension.
- Write a Python program to cluster list elements based on their remainder when divided by a given number.
- Write a Python program to use itertools.groupby to group sorted list elements by a custom function.
Python Code Editor:
Previous: Write a Python program to combines two or more dictionaries, creating a list of values for each key.
Next: Write a Python program to map the values of a given list to a dictionary using a function, where the key-value pairs consist of the original value as the key and the result of the function as the value.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics