Python: Check if a specific Key and a value exist in a dictionary
Write a Python program to check if a specific key and a value exist in a dictionary.
Visual Presentation:
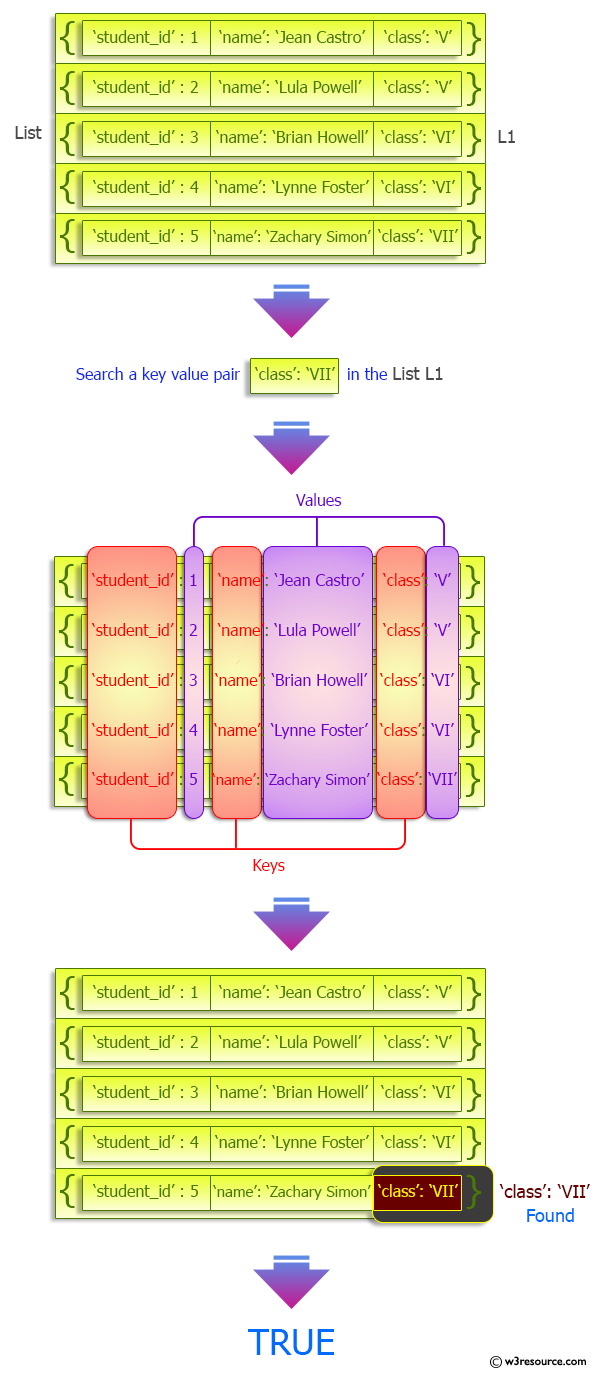
Sample Solution:
Python Code:
# Define a function 'test' that takes a list of dictionaries 'dictt', a 'key', and a 'value' as arguments.
def test(dictt, key, value):
# Check if any sub-dictionary in the list 'dictt' has the given 'key' with the specified 'value'.
if any(sub[key] == value for sub in dictt):
return True # If found, return True.
return False # If not found, return False.
# Create a list of dictionaries 'students' with student details.
students = [
{'student_id': 1, 'name': 'Jean Castro', 'class': 'V'},
{'student_id': 2, 'name': 'Lula Powell', 'class': 'V'},
{'student_id': 3, 'name': 'Brian Howell', 'class': 'VI'},
{'student_id': 4, 'name': 'Lynne Foster', 'class': 'VI'},
{'student_id': 5, 'name': 'Zachary Simon', 'class': 'VII'}
]
# Print a message indicating the start of the code section and display the original dictionary.
print("\nOriginal dictionary:")
print(students)
# Print a message indicating the purpose and demonstrate the 'test' function's usage
# to check if specific keys and values exist in the dictionary.
print("\nCheck if a specific Key and a value exist in the said dictionary:")
print(test(students, 'student_id', 1))
print(test(students, 'name', 'Brian Howell'))
print(test(students, 'class', 'VII'))
print(test(students, 'class', 'I'))
print(test(students, 'name', 'Brian Howelll'))
print(test(students, 'student_id', 11))
Sample Output:
Original dictionary: [{'student_id': 1, 'name': 'Jean Castro', 'class': 'V'}, {'student_id': 2, 'name': 'Lula Powell', 'class': 'V'}, {'student_id': 3, 'name': 'Brian Howell', 'class': 'VI'}, {'student_id': 4, 'name': 'Lynne Foster', 'class': 'VI'}, {'student_id': 5, 'name': 'Zachary Simon', 'class': 'VII'}] Check if a specific Key and a value exist in the said dictionary: True True True False False False
Flowchart:
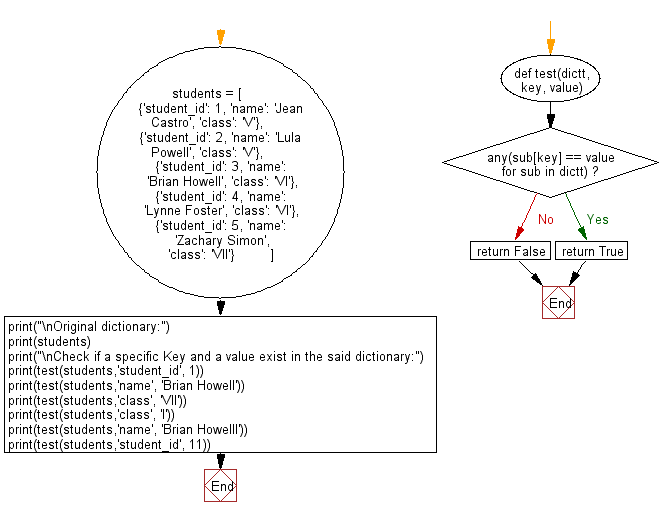
Python Code Editor:
Previous: Write a Python program to get the total length of all values of a given dictionary with string values.
Next: Write a Python program to invert a given dictionary with non-unique hashable values.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics