Python: Convert a given dictionary into a list of lists
Write a Python program to convert a dictionary into a list of lists.
Sample Solution:
Python Code:
# Define a function 'test' that converts a dictionary into a list of lists containing key-value pairs.
def test(dictt):
# Use the 'items' method to get key-value pairs, then convert it to a list of lists.
result = list(map(list, dictt.items()))
return result
# Create a dictionary 'color_dict' with numeric keys and corresponding color names as values.
color_dict = {1 : 'red', 2 : 'green', 3 : 'black', 4 : 'white', 5 : 'black'}
# Print a message indicating the start of the code section and the dictionary being processed.
print("\nOriginal Dictionary:")
print(color_dict)
# Call the 'test' function to convert the dictionary into a list of lists and print the result.
print("Convert the said dictionary into a list of lists:")
print(test(color_dict))
# Create another dictionary 'color_dict' with string keys and corresponding names as values.
color_dict = {'1' : 'Austin Little', '2' : 'Natasha Howard', '3' : 'Alfred Mullins', '4' : 'Jamie Rowe'}
# Print a message indicating the start of the code section and the new dictionary being processed.
print("\nOriginal Dictionary:")
print(color_dict)
# Call the 'test' function to convert the dictionary into a list of lists and print the result.
print("Convert the said dictionary into a list of lists:")
print(test(color_dict))
Sample Output:
Original Dictionary: {1: 'red', 2: 'green', 3: 'black', 4: 'white', 5: 'black'} Convert the said dictionary into a list of lists: [[1, 'red'], [2, 'green'], [3, 'black'], [4, 'white'], [5, 'black']] Original Dictionary: {'1': 'Austin Little', '2': 'Natasha Howard', '3': 'Alfred Mullins', '4': 'Jamie Rowe'} Convert the said dictionary into a list of lists: [['1', 'Austin Little'], ['2', 'Natasha Howard'], ['3', 'Alfred Mullins'], ['4', 'Jamie Rowe']]
Flowchart:
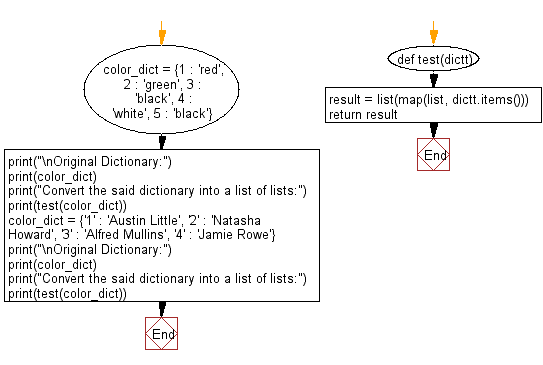
Python Code Editor:
Previous: Write a Python program to access dictionary key's element by index.
Next: Write a Python program to filter even numbers from a given dictionary values.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics