Python: Extract a list of values from a given list of dictionaries
52. Extract List of Values from List of Dictionaries
Write a Python program to extract a list of values from a given list of dictionaries.
Visual Presentation:
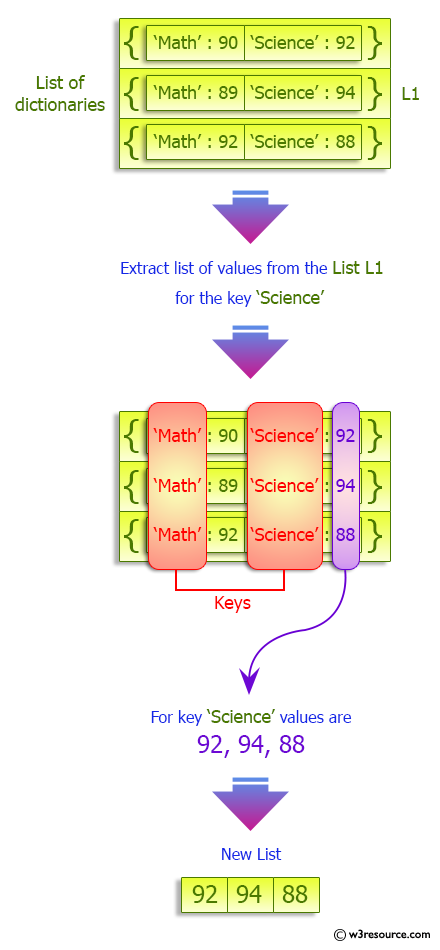
Sample Solution:
Python Code:
# Define a function 'test' that extracts a list of values for a specific subject ('marks') from a list of dictionaries ('lst').
def test(lst, marks):
# Use a list comprehension to extract the values associated with the subject ('marks') in each dictionary.
result = [d[marks] for d in lst if marks in d]
return result
# Create a list of dictionaries 'marks' with subjects 'Math' and 'Science' and associated marks.
marks = [{'Math': 90, 'Science': 92},
{'Math': 89, 'Science': 94},
{'Math': 92, 'Science': 88}]
# Print a message indicating the start of the code section and the original list of dictionaries.
print("\nOriginal Dictionary:")
print(marks)
# Define the subject 'subj' as "Science".
subj = "Science"
# Print a message indicating the intention to extract values for the subject 'subj' from the list of dictionaries.
print("\nExtract a list of values from the said list of dictionaries where subject =", subj)
# Call the 'test' function to extract the values associated with the subject 'subj' and print the result.
print(test(marks, subj))
# Print a message indicating the start of the code section and the original list of dictionaries.
print("\nOriginal Dictionary:")
print(marks)
# Define the subject 'subj' as "Math".
subj = "Math"
# Print a message indicating the intention to extract values for the subject 'subj' from the list of dictionaries.
print("\nExtract a list of values from the said list of dictionaries where subject =", subj)
# Call the 'test' function to extract the values associated with the subject 'subj' and print the result.
print(test(marks, subj))
Sample Output:
Original Dictionary: [{'Math': 90, 'Science': 92}, {'Math': 89, 'Science': 94}, {'Math': 92, 'Science': 88}] Extract a list of values from said list of dictionaries where subject = Science [92, 94, 88] Original Dictionary: [{'Math': 90, 'Science': 92}, {'Math': 89, 'Science': 94}, {'Math': 92, 'Science': 88}] Extract a list of values from said list of dictionaries where subject = Math [90, 89, 92]
Flowchart:
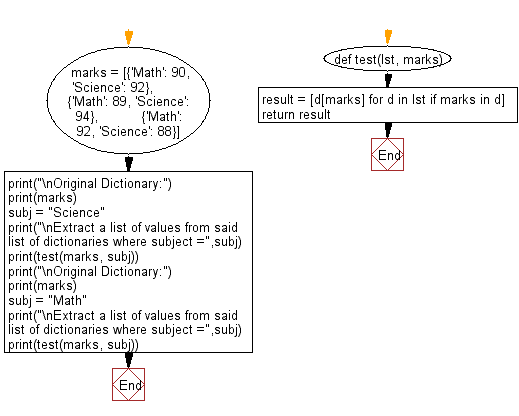
For more Practice: Solve these Related Problems:
- Write a Python program to extract the values for a specified key from a list of dictionaries using list comprehension.
- Write a Python program to iterate through a list of dictionaries and collect values corresponding to a given key, handling missing keys.
- Write a Python program to use the map() function to retrieve values for a specified key from each dictionary in a list.
- Write a Python function that returns a list of values for a given key from a list of dictionaries, excluding dictionaries where the key is absent.
Go to:
Previous: A Python Dictionary contains List as value. Write a Python program to update the list values in the said dictionary.
Next: Write a Python program to find the length of a given dictionary values.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.