Python: Drop empty Items from a given Dictionary
41. Drop Empty Items from Dictionary
Write a Python program to drop empty items from a given dictionary.
Visual Presentation:
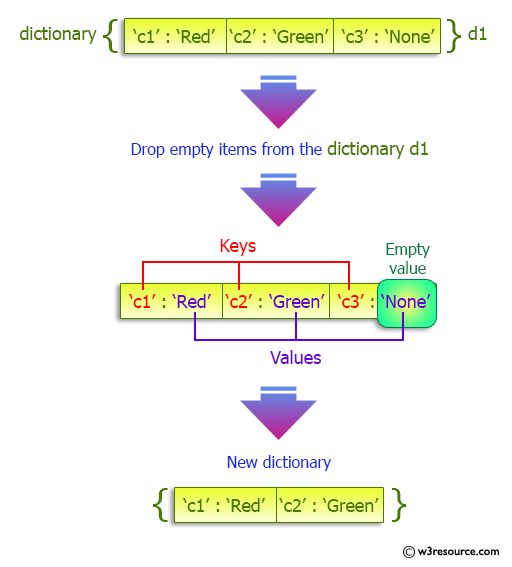
Sample Solution:
Python Code:
# Create a dictionary 'dict1' with keys and associated values, including a 'None' value.
dict1 = {'c1': 'Red', 'c2': 'Green', 'c3': None}
# Print a message indicating the start of the code section and the original dictionary.
print("Original Dictionary:")
print(dict1)
# Print a message indicating the intention to create a new dictionary without empty items.
print("New Dictionary after dropping empty items:")
# Use a dictionary comprehension to create a new dictionary that excludes items with 'None' values.
# Iterate through the key-value pairs in 'dict1' and include them in the new dictionary if the value is not 'None'.
dict1 = {key: value for (key, value) in dict1.items() if value is not None}
# Print the new dictionary containing only non-empty items.
print(dict1)
Sample Output:
Original Dictionary: {'c1': 'Red', 'c2': 'Green', 'c3': None} New Dictionary after dropping empty items: {'c1': 'Red', 'c2': 'Green'}
Flowchart:
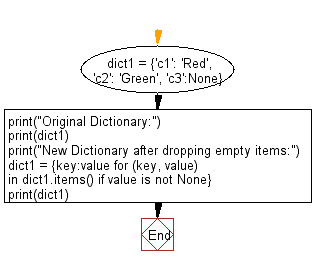
For more Practice: Solve these Related Problems:
- Write a Python program to remove keys with values that are None, empty strings, or empty lists from a dictionary using dictionary comprehension.
- Write a Python program to filter out falsey values (e.g., None, "", [], {}) from a dictionary and return a new dictionary.
- Write a Python function to recursively drop empty items from a nested dictionary.
- Write a Python program to iterate over a dictionary and remove keys whose values evaluate to False.
Go to:
Previous: Write a Python program to create a dictionary of keys x, y, and z where each key has as value a list from 11-20, 21-30, and 31-40 respectively. Access the fifth value of each key from the dictionary.
Next: Write a Python program to filter a dictionary based on values.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.