Python: Check whether a given key already exists in a dictionary
4. Check Key Existence in Dictionary
Write a Python program to check whether a given key already exists in a dictionary.
Sample Solution-1:
Python Code:
# Create a dictionary 'd' with key-value pairs.
d = {1: 10, 2: 20, 3: 30, 4: 40, 5: 50, 6: 60}
# Define a function 'is_key_present' that takes an argument 'x'.
def is_key_present(x):
# Check if 'x' is a key in the dictionary 'd'.
if x in d:
# If 'x' is present in 'd', print a message indicating that the key is present.
print('Key is present in the dictionary')
else:
# If 'x' is not present in 'd', print a message indicating that the key is not present.
print('Key is not present in the dictionary')
# Call the 'is_key_present' function with the argument 5 to check if 5 is a key in the dictionary.
is_key_present(5)
# Call the 'is_key_present' function with the argument 9 to check if 9 is a key in the dictionary.
is_key_present(9)
Sample Output:
Key is present in the dictionary Key is not present in the dictionary
Flowchart:
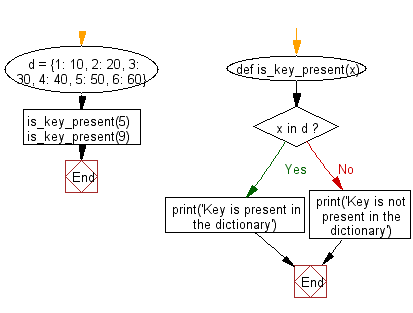
Sample Solution-2:
Python Code:
# Define a function 'key_in_dict' that takes a dictionary 'd' and a 'key' as arguments.
# It checks if the 'key' is present in the dictionary 'd' and returns a boolean value.
def key_in_dict(d, key):
return (key in d)
# Create a dictionary 'students' with key-value pairs.
students = {
'Theodore': 19,
'Roxanne': 22,
'Mathew': 21,
'Betty': 20
}
# Print a message indicating the start of the code section.
print("\nOriginal dictionary elements:")
# Print the original dictionary 'students'.
print(students)
# Call the 'key_in_dict' function to check if the key 'Roxanne' is present in the 'students' dictionary.
# Print the result, which is a boolean value.
print(key_in_dict(students, 'Roxanne'))
# Call the 'key_in_dict' function to check if the key 'Gina' is present in the 'students' dictionary.
# Print the result, which is a boolean value.
print(key_in_dict(students, 'Gina'))
Sample Output:
Original dictionary elements: {'Theodore': 19, 'Roxanne': 22, 'Mathew': 21, 'Betty': 20} True False
Flowchart:
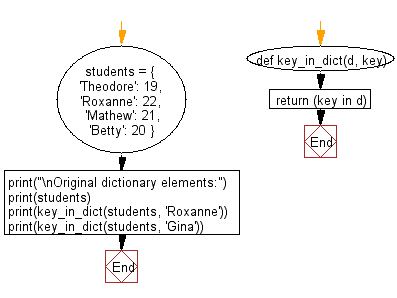
For more Practice: Solve these Related Problems:
- Write a Python script to check if a given key exists in a dictionary using the in operator.
- Write a Python script to determine key existence by iterating through the dictionary keys.
- Write a Python script to use the get() method to check for a key and return a default value if it is missing.
- Write a Python script to implement a function that returns True if a key exists in a dictionary and False otherwise.
Go to:
Previous: Write a Python program to concatenate following dictionaries to create a new one.
Next: Write a Python program to iterate over dictionaries using for loops.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.