Python: Create a dictionary from two lists without losing duplicate values
36. Create Dictionary from Two Lists Without Losing Duplicates
Write a Python program to create a dictionary from two lists without losing duplicate values.
Visual Presentation:
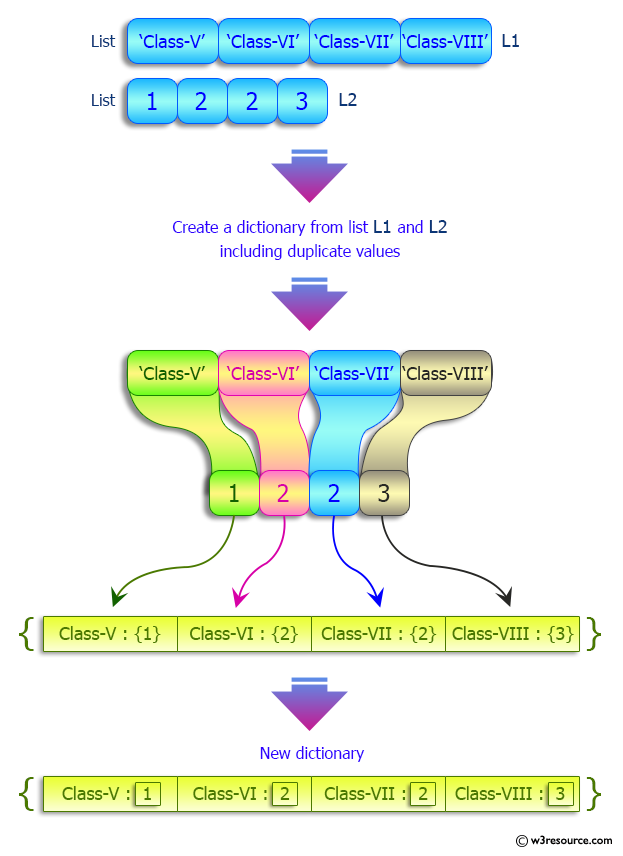
Sample Solution:
Python Code:
# Import the 'defaultdict' class from the 'collections' module.
from collections import defaultdict
# Create a list 'class_list' with class names and a list 'id_list' with corresponding IDs.
class_list = ['Class-V', 'Class-VI', 'Class-VII', 'Class-VIII']
id_list = [1, 2, 2, 3]
# Create a defaultdict 'temp' with set as the default factory function.
temp = defaultdict(set)
# Iterate through paired elements of 'class_list' and 'id_list' using the 'zip' function.
for c, i in zip(class_list, id_list):
# Add the 'i' value to the set associated with the 'c' key in the 'temp' defaultdict.
temp[c].add(i)
# Print the 'temp' defaultdict, which groups IDs by class name.
print(temp)
Sample Output:
defaultdict(<class 'set'>, {'Class-V': {1}, 'Class-VI': {2}, 'Class-VII': {2}, 'Class-VIII': {3}})
For more Practice: Solve these Related Problems:
- Write a Python program to convert two lists into a dictionary where keys map to a set of values to preserve duplicates.
- Write a Python program to use collections.defaultdict(set) to merge two lists into a dictionary without losing duplicate values.
- Write a Python program to iterate over two lists and store duplicate values in a set within the dictionary for each key.
- Write a Python program to implement a function that creates a dictionary from two lists, ensuring duplicate values are stored in a list or set.
Python Code Editor:
Previous: Write a Python program to sort Counter by value.
Next: Write a Python program to replace dictionary values with their sum.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics