Implementing a Python decorator for function results caching
4. Implement a Decorator to Cache Function Results
Write a Python program that implements a decorator to cache the result of a function.
Sample Solution:
Python Code:
def cache_result(func):
cache = {}
def wrapper(*args, **kwargs):
key = (*args, *kwargs.items())
if key in cache:
print("Retrieving result from cache...")
return cache[key]
result = func(*args, **kwargs)
cache[key] = result
return result
return wrapper
# Example usage
@cache_result
def calculate_multiply(x, y):
print("Calculating the product of two numbers...")
return x * y
# Call the decorated function multiple times
print(calculate_multiply(4, 5)) # Calculation is performed
print(calculate_multiply(4, 5)) # Result is retrieved from cache
print(calculate_multiply(5, 7)) # Calculation is performed
print(calculate_multiply(5, 7)) # Result is retrieved from cache
print(calculate_multiply(-3, 7)) # Calculation is performed
print(calculate_multiply(-3, 7)) # Result is retrieved from cache
Sample Output:
Calculating the product of two numbers... 20 Retrieving result from cache... 20 Calculating the product of two numbers... 35 Retrieving result from cache... 35 Calculating the product of two numbers... -21 Retrieving result from cache... -21
Explanation:
In the above exercise,
The function "calculate_multiply()" is decorated with '@cache_result'. When 'calculate_multiply' is called with the same arguments multiple times, the decorator checks the cache to see if the result is already available. If it is, the cached result is returned, and if not, the calculation is performed and the result is stored in the cache.
The example code output demonstrates the caching behavior. The first call to calculate_multiply(4, 5) performs the calculation and prints "Calculating the product of two numbers...". The second call with the same arguments retrieves the result from the cache and prints "Retrieving result from cache...". The same pattern applies to subsequent calls with the same or different arguments.
Flowchart:
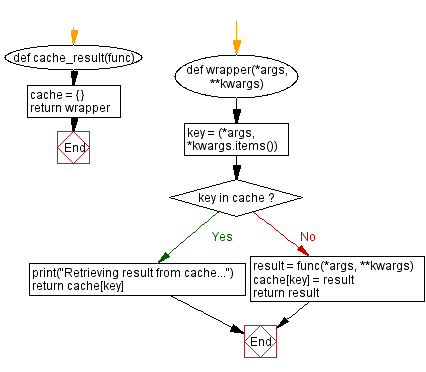
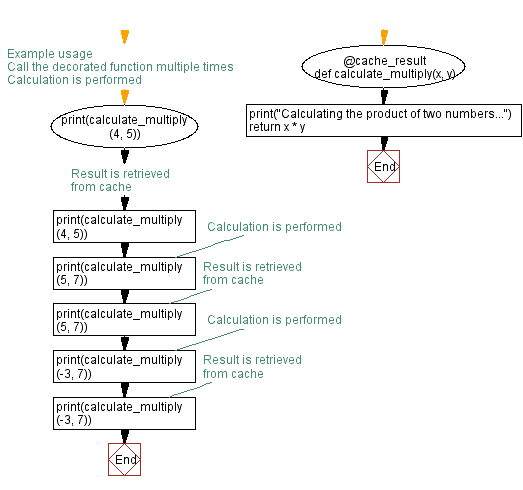
For more Practice: Solve these Related Problems:
- Write a Python decorator that caches a function’s return values using a dictionary keyed by its arguments.
- Write a Python decorator that implements an LRU cache for a function, evicting the least recently used entries when capacity is exceeded.
- Write a Python decorator that stores results of expensive function calls and returns the cached result on subsequent calls with identical arguments.
- Write a Python decorator that clears the cache after a specific time interval (expiration), ensuring fresh results periodically.
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Creating a Python decorator to convert function return value.
Next: Implementing a Python decorator for argument validation.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.