Python: Calculate an age in year
39. Age Calculator
Write a Python program to calculate an age in years.
Sample Solution:
Python Code:
# Import the date class from datetime module
from datetime import date
# Define a function called calculate_age which calculates the age based on the date of birth
def calculate_age(dtob):
# Get the current date
today = date.today()
# Calculate the age by subtracting the birth year from the current year
# Adjust the age if the birth month and day are after today's month and day
return today.year - dtob.year - ((today.month, today.day) < (dtob.month, dtob.day))
# Print an empty line
print()
# Print the age calculated based on the date of birth October 12, 2006
print(calculate_age(date(2006,10,12)))
# Print the age calculated based on the date of birth January 12, 1989
print(calculate_age(date(1989,1,12)))
# Print an empty line
print()
Output:
10 28
Explanation:
In the exercise above,
- The code imports the "date" class from the "datetime" module.
- It defines a function named "calculate_age()" which calculates the age based on the provided date of birth (dtob).
- Inside the "calculate_age()" function:
- It gets the current date using "date.today()" function.
- It calculates age by subtracting the birth year from the current year.
- It adjusts the age if the birth month and day are after today's month and day.
- It calculates and prints the age based on the date of birth October 12, 2006, by calling the "calculate_age()" function.
- It calculates and prints the age based on the date of birth January 12, 1989, by calling the "calculate_age()" function.
Flowchart:
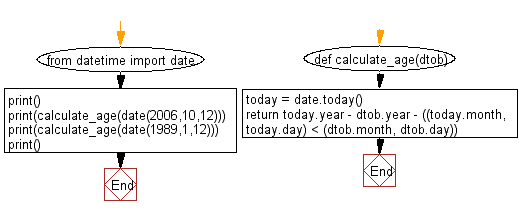
For more Practice: Solve these Related Problems:
- Write a Python program to calculate a person's age in years given their birthdate and the current date.
- Write a Python script that computes age from a date of birth and then determines if the person is eligible for a senior discount (age 65+).
- Write a Python function to calculate age in years, months, and days given a birthdate and then format the output as a detailed string.
- Write a Python program to determine age in years from a given birthdate and compare it with another age to check for equality.
Go to:
Previous: Write a Python program to get last modified information of a file.
Next: Write a Python program to get the current date time information.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.