Python: Convert two date difference in days, hours, minutes, seconds
Write a Python program to convert difference of two dates into days, hours, minutes, and seconds.
Sample Solution:
Python Code:
# Import the datetime and time classes from datetime module
from datetime import datetime, time
# Define a function called date_diff_in_seconds which calculates the difference in seconds between two datetime objects
def date_diff_in_seconds(dt2, dt1):
# Calculate the time difference between dt2 and dt1
timedelta = dt2 - dt1
# Return the total time difference in seconds
return timedelta.days * 24 * 3600 + timedelta.seconds
# Define a function called dhms_from_seconds which converts seconds into days, hours, minutes, and seconds
def dhms_from_seconds(seconds):
# Calculate minutes and remaining seconds
minutes, seconds = divmod(seconds, 60)
# Calculate hours and remaining minutes
hours, minutes = divmod(minutes, 60)
# Calculate days and remaining hours
days, hours = divmod(hours, 24)
# Return days, hours, minutes, and seconds
return (days, hours, minutes, seconds)
# Specified date: January 1, 2015, at 01:00:00
date1 = datetime.strptime('2015-01-01 01:00:00', '%Y-%m-%d %H:%M:%S')
# Current date and time
date2 = datetime.now()
# Print the time difference in days, hours, minutes, and seconds between the current date and the specified date
print("\n%d days, %d hours, %d minutes, %d seconds" % dhms_from_seconds(date_diff_in_seconds(date2, date1)))
# Print an empty line
print()
Output:
858 days, 12 hours, 34 minutes, 21 seconds
Explanation:
In the exercise above,
- The code imports the "datetime" and "time" classes from the "datetime" module.
- It defines a function named "date_diff_in_seconds()" which calculates the difference in seconds between two "datetime" objects.
- Inside the "date_diff_in_seconds()" function:
- It calculates the time difference (timedelta) between 'dt2' and 'dt1' using simple subtraction.
- It converts the time difference into seconds by multiplying the number of days by 24 hours and then by 3600 seconds, and adding the number of seconds in the 'timedelta'.
- It defines a function named "dhms_from_seconds()" which converts seconds into days, hours, minutes, and seconds.
- Inside the "dhms_from_seconds()" function:
- It uses the "divmod()" function to calculate minutes, hours, and days from the given number of seconds.
- It defines 'date1' as a specified date, January 1, 2015, at 01:00:00, using the "strptime()" function to parse the string into a "datetime" object.
- It defines 'date2' as the current date and time using the "datetime.now()" function.
- It prints the time difference in days, hours, minutes, and seconds between 'date2' and 'date1'.
Flowchart:
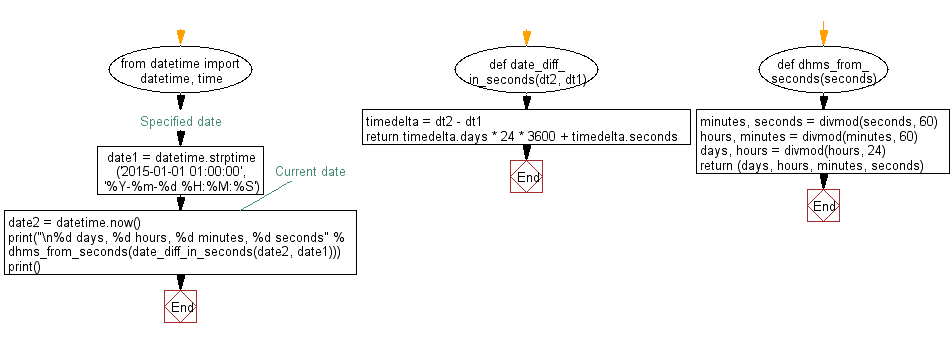
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to calculate two date difference in seconds.
Next: Write a Python program to get last modified information of a file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics