Python: Convert a date to Unix timestamp
35. Date to Unix Timestamp
Write a Python program to convert a date to a Unix timestamp.
Sample Solution:
Python Code:
# Import the datetime module
import datetime
# Import the time module
import time
# Create a datetime object representing February 25, 2016, at 23:23 (11:23 PM)
dt = datetime.datetime(2016, 2, 25, 23, 23)
# Print an empty line
print()
# Print the Unix timestamp corresponding to the datetime object 'dt'
print("Unix Timestamp: ",(time.mktime(dt.timetuple())))
# Print an empty line
print()
Output:
Unix Timestamp: 1456422780.0
Explanation:
In the exercise above,
- The code imports the "datetime" module, which provides classes for manipulating dates and times.
- It also imports the "time" module, which provides functions for working with time-related tasks.
- It creates a "datetime" object named "dt" representing the date and time February 25, 2016, at 11:23 PM (23:23).
- It calculates the Unix timestamp corresponding to the "datetime" object "dt" using the "mktime()" function from the "time" module, which converts a "struct_time" object to a Unix timestamp.
- It prints the Unix timestamp along with a descriptive string indicating what it represents.
Flowchart:
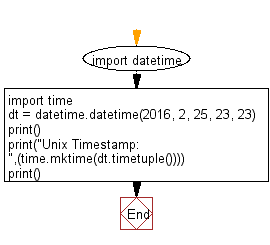
For more Practice: Solve these Related Problems:
- Write a Python program to convert a given date in "MM/DD/YYYY" format to its Unix timestamp and then validate the conversion.
- Write a Python script to accept a date, convert it to a datetime object, and then output the Unix timestamp in seconds.
- Write a Python function to convert multiple date strings to Unix timestamps and then print them in ascending order.
- Write a Python program to transform a date into a Unix timestamp and then convert it back to verify the original date.
Go to:
Previous: Write a Python program to display the date and time in a human-friendly string.
Next: Write a Python program to calculate two date difference in seconds.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.