Python: Get the GMT and local current time
29. GMT and Local Time
Write a Python program to get GMT and the local time.
Sample Solution:
Python Code:
# Import the gmtime and strftime functions from the time module
from time import gmtime, strftime
# Import the time module
import time
# Print the current time in GMT (Greenwich Mean Time) format
print("\nGMT: " + time.strftime("%a, %d %b %Y %I:%M:%S %p %Z", time.gmtime()))
# Print the current time in local time zone format
# The strftime function is used directly since it was imported earlier
print("Local: " + strftime("%a, %d %b %Y %I:%M:%S %p %Z\n"))
Output:
GMT: Mon, 08 May 2017 06:21:07 AM GMT Local: Mon, 08 May 2017 11:51:07 AM IST
Explanation:
In the exercise above,
- The code imports the "gmtime" and "strftime" functions from the "time" module and the entire "time" module separately.
- Print the current time in different time zones:
- It prints the current time in GMT (Greenwich Mean Time) format using the "strftime()" function with the '%Z' directive to display the time zone.
- "time.gmtime()" retrieves the current time in GMT.
- It prints the current time in the local time zone format using the "strftime()" function.
- Formatting Time Strings:
- The "strftime()" function is used to format time strings according to the specified format directives, such as '%a' for abbreviated weekday name, '%d' for day of the month, '%b' for abbreviated month name, '%Y' for year, '%I' for hour (12-hour clock), '%M' for minute, '%S' for second, '%p' for AM/PM, and '%Z' for time zone name.
- Finally the formatted time strings are printed to the console.
Flowchart:
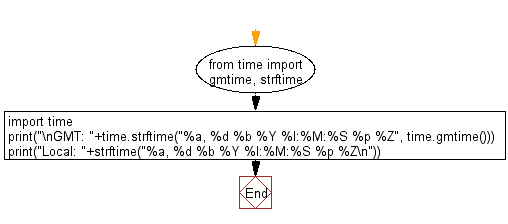
For more Practice: Solve these Related Problems:
- Write a Python program to display both the local time and GMT time in "HH:MM:SS" format and then calculate the time difference.
- Write a Python script that retrieves the current local time and GMT time, then formats both with timezone labels.
- Write a Python program to print the current local time and its corresponding GMT time and then compare their hour components.
- Write a Python function to display local and GMT times and then output which one is ahead based on the timezone offset.
Go to:
Previous: Write a Python program to get the dates 30 days before and after from the current date.
Next: Write a Python program convert a date to timestamp.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.