Python: Print a string five times, delay three seconds
25. Delayed String Printer
Write a Python program to print a string five times, with a delay of three seconds.
Sample Solution:
Python Code:
# Import the time module
import time
# Initialize a variable 'x' with value 0
x = 0
# Print a newline character followed by a message indicating a delay
print("\nw3resource will print five times, with a delay of three seconds each.")
# Execute a loop that repeats until 'x' is less than 5
while x < 5:
# Print the string "w3resource"
print("w3resource")
# Pause the execution of the program for three seconds
time.sleep(3)
# Increment the value of 'x' by 1
x = x + 1
Output:
w3resource will print five times, delay for three seconds. w3resource w3resource w3resource w3resource w3resource
Explanation:
In the exercise above,
- The code imports the "time" module.
- Initializing variables:
- It initializes a variable 'x' with an initial value of 0.
- Printing Message: It prints a message indicating that "w3resource" will be printed five times with a delay of three seconds between each print. This is achieved by using the "print()" function with the newline character '\n' to create space before the message.
- Looping and Printing:
- It enters a while loop that continues until the value of 'x' is less than 5.
- Within the loop, it prints the string "w3resource" using the "print()" function.
- It then pauses the program execution for three seconds using the "time.sleep()" function to introduce a delay.
- After the delay, it increments the value of 'x' by 1.
- Execution and termination:
- Once the loop finishes executing (after "w3resource" has been printed five times), the program terminates.
Flowchart:
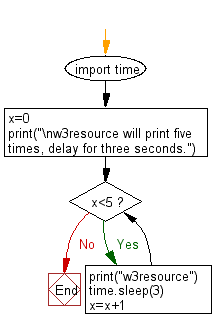
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to count the number of Monday of the 1st day of the month from 2015 to 2016.
Next: Write a Python program calculates the date six months from the current date using the datetime module.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics