Python: Add year(s) with a given date and display the new date
16. Add Years to Date
Write a Python program to add year(s) to a given date and display the updated date.
Sample Solution:
Python Code:
# Import the datetime module
import datetime
# Import the date class from the datetime module
from datetime import date
# Define a function named addYears that takes a date (d) and a number of years (years) as input
def addYears(d, years):
# Try to return the same day of the current year with the year adjusted by 'years'
try:
# If the same day is possible in the adjusted year, return it
return d.replace(year=d.year + years)
# If adjusting the year causes a ValueError (e.g., trying to go from February 29 to March 1 in a non-leap year)
except ValueError:
# Calculate and return the closest possible date by adding the difference between the adjusted year's January 1st and the original year's January 1st
return d + (date(d.year + years, 1, 1) - date(d.year, 1, 1))
# Print the result of adding -1 year to January 1st, 2015
print(addYears(datetime.date(2015, 1, 1), -1))
# Print the result of adding 0 years to January 1st, 2015
print(addYears(datetime.date(2015, 1, 1), 0))
# Print the result of adding 2 years to January 1st, 2015
print(addYears(datetime.date(2015, 1, 1), 2))
# Print the result of adding 1 year to February 29th, 2000 (a leap year)
print(addYears(datetime.date(2000, 2, 29), 1))
Output:
2014-01-01 2015-01-01 2017-01-01 2001-03-01
Explanation:
In the exercise above,
- The code imports the "datetime" module, which provides functionalities to work with dates and times. It also imports the "date" class from the "datetime" module.
- Define the function to add years to a date:
- The code defines a function named "addYears()" that takes a date (d) and a number of years (years) as input.
- Added years of functionality:
- The function attempts to return the same day of the year in an adjusted year by replacing the year of the input date (d) with the adjusted year (d.year + years). If this adjustment results in a valid date, it returns that date.
- If adjusting the year causes a 'ValueError' (e.g., attempting to move from February 29th to March 1st in a non-leap year), it handles this case by calculating and returning the closest possible date. This is done by adding the difference between January 1st of the adjusted year and January 1st of the original year to the input date.
- Finally it prints the results of adding different numbers of years to specific dates, demonstrating the functionality of the "addYears()" function.
Flowchart:
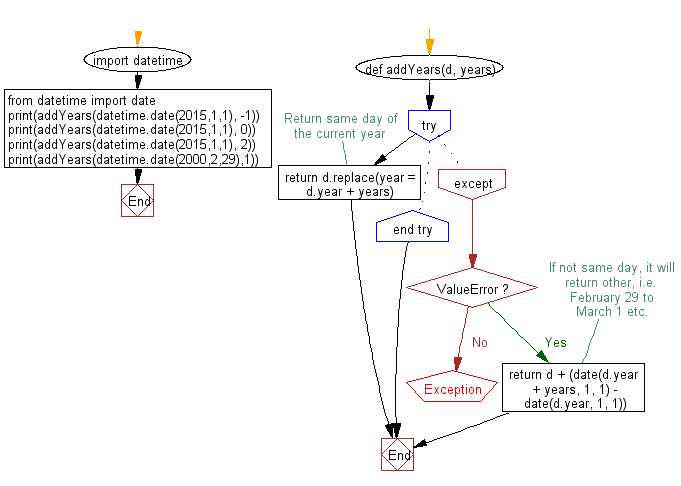
For more Practice: Solve these Related Problems:
- Write a Python program to add or subtract a specified number of years to a given date and then adjust for leap day discrepancies.
- Write a Python function that takes a date and a number of years, and returns the new date while handling February 29 appropriately.
- Write a Python script to compute the updated date after adding a positive or negative number of years and then format the result as "MM/DD/YYYY".
- Write a Python program to update a given date by adding years and then check if the new date falls on a weekend.
Go to:
Previous: Write a Python program to select all the Sundays of a specified year.
Next: Write a Python program to drop microseconds from datetime.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.