Python: Convert Year/Month/Day to Day of Year
11. Day of Year Converter
Write a Python program to convert Year/Month/Day to Day of Year in Python.
Sample Solution:
Python Code:
# Import the datetime module
import datetime
# Get the current date and time and assign it to the variable 'today'
today = datetime.datetime.now()
# Calculate the day of the year by subtracting January 1st of the current year from the current date and time,
# then retrieve the number of days and add 1 (since January 1st should be considered as day 1)
day_of_year = (today - datetime.datetime(today.year, 1, 1)).days + 1
# Print the calculated day of the year
print(day_of_year)
Output:
126
Explanation:
In the exercise above,
- The code imports the datetime module, which provides functionalities to work with dates and times.
- Get the current date and time:
- It retrieves the current date and time using "datetime.datetime.now()" and assigns it to the variable 'today'.
- Calculating the day of the year:
- It calculates the day of the year by subtracting January 1st of the current year from the current date and time. This effectively gives the number of days elapsed since the start of the year.
- It retrieves the number of days from the resulting timedelta object and adds 1 to account for January 1st, which should be considered as day 1.
- Finally it prints the calculated day of the year using "print(day_of_year)".
Flowchart:
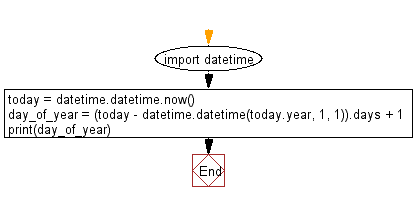
For more Practice: Solve these Related Problems:
- Write a Python program to convert a given date (YYYY-MM-DD) to its corresponding day of the year and then print the result.
- Write a Python script that takes a date as input and returns both the day of the year and the remaining days in that year.
- Write a Python function to calculate the day number of the year for multiple dates and then output the maximum and minimum values.
- Write a Python program to convert a list of dates to their respective day of year numbers and then sort the list based on these numbers.
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to add 5 seconds with the current time.
Next: Write a Python program to get current time in milliseconds.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.