Python: Display the various Date Time formats
Write a Python script to display the various Date Time formats.
a) Current date and time
b) Current year
c) Month of year
d) Week number of the year
e) Weekday of the week
f) Day of year
g) Day of the month
h) Day of week
Sample Solution:
Python Code:
# Import the time module
import time
# Import the datetime module
import datetime
# Print the current date and time using datetime
print("Current date and time: " , datetime.datetime.now())
# Print the current year extracted from today's date
print("Current year: ", datetime.date.today().strftime("%Y"))
# Print the month of the year extracted from today's date
print("Month of year: ", datetime.date.today().strftime("%B"))
# Print the week number of the year extracted from today's date
print("Week number of the year: ", datetime.date.today().strftime("%W"))
# Print the weekday of the week extracted from today's date
print("Weekday of the week: ", datetime.date.today().strftime("%w"))
# Print the day of the year extracted from today's date
print("Day of year: ", datetime.date.today().strftime("%j"))
# Print the day of the month extracted from today's date
print("Day of the month : ", datetime.date.today().strftime("%d"))
# Print the day of the week extracted from today's date
print("Day of week: ", datetime.date.today().strftime("%A"))
Output:
Current date and time: 2017-05-05 17:21:19.106836 Current year: 2017 Month of year: May Week number of the year: 18 Weekday of the week: 5 Day of year: 125 Day of the month : 05 Day of week: Friday
Explanation:
In the exercise above,
- The code imports two modules: "time" and "datetime".
- It then prints various pieces of information about the current date and time using the "datetime" module:
- The current date and time are printed using "datetime.datetime.now()".
- The current year is extracted from today's date and printed.
- The month of the year is extracted from today's date and printed.
- The week number of the year is extracted from today's date and printed.
- The weekday of the week is extracted from today's date and printed.
- The day of the year is extracted from today's date and printed.
- The day of the month is extracted from today's date and printed.
- The day of the week is extracted from today's date and printed.
- The "strftime()" method is used to format the date and time components according to specific format codes.
Flowchart:
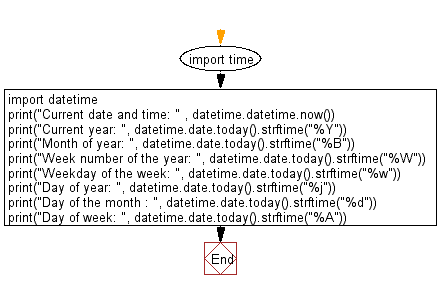
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Datetime Exercise Home.
Next: Write a Python program to determine whether a given year is a leap year.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics