Python: Sort a list of elements using Selection sort
20. Selection Sort (Repeat)
Write a Python program to sort a list of elements using Selection sort.
According to Wikipedia "In computer science, selection sort is a sorting algorithm, specifically an in-place comparison sort. It has O(n2) time complexity, making it inefficient on large lists, and generally performs worse than the similar insertion sort".
Sample Solution:
Python Code:
def selection_sort(nums):
for i, n in enumerate(nums):
mn = min(range(i,len(nums)), key=nums.__getitem__)
nums[i], nums[mn] = nums[mn], n
return nums
user_input = input("Input numbers separated by a comma:\n").strip()
nums = [int(item) for item in user_input.split(',')]
print(selection_sort(nums))
Sample Output:
Input numbers separated by a comma: 15, 79, 25, 37, 68 [15, 25, 37, 68, 79]
Flowchart:
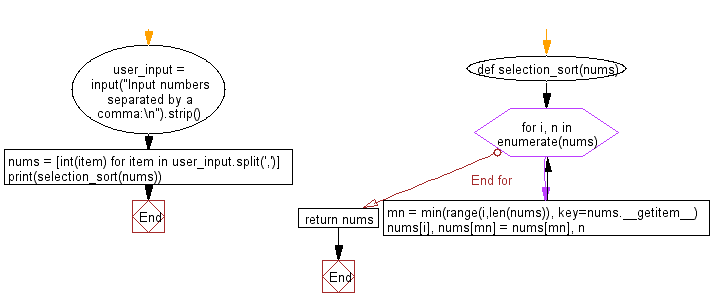
For more Practice: Solve these Related Problems:
- Write a Python program to implement selection sort and output the minimum element found during each pass.
- Write a Python script to perform selection sort on a list and highlight the index swaps in the output.
- Write a Python program to implement selection sort on a list of dictionaries by selecting the minimum value for a given key.
- Write a Python function to implement selection sort and then print the partially sorted list after every iteration.
Go to:
Previous: Write a Python program to sort a list of elements using Radix sort.
Next: Write a Python program to sort a list of elements using Time sort.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.