Python Data Structures and Algorithms - Recursion: List sum
Write a Python program to sum recursion lists using recursion.
Sample Solution:
Python Code:
# Define a function named recursive_list_sum that calculates the sum of elements in a nested list
def recursive_list_sum(data_list):
# Initialize a variable 'total' to store the cumulative sum
total = 0
# Iterate through each element in the input list
for element in data_list:
# Check if the current element is a list (nested list)
if type(element) == type([]):
# If the element is a list, recursively call the recursive_list_sum function on the element
total = total + recursive_list_sum(element)
else:
# If the element is not a list, add its value to the total
total = total + element
# Return the total sum
return total
# Print the result of calling the recursive_list_sum function with the input list [1, 2, [3,4], [5,6]]
print(recursive_list_sum([1, 2, [3, 4], [5, 6]]))
Sample Output:
21
Flowchart:
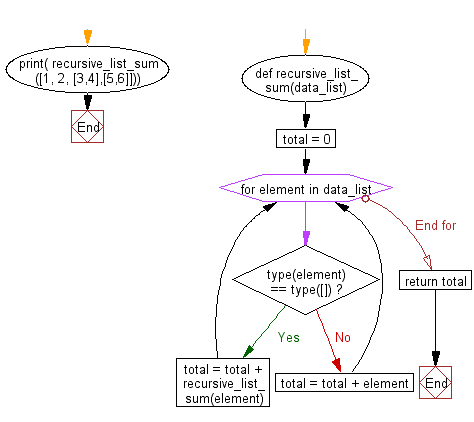
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to converting an integer to a string in any base.
Next: Write a Python program to get the factorial of a non-negative integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics