Python Linked List: Search a specific item in a singly linked list and return true if the item is found otherwise return false
3. Search in Singly Linked List
Write a Python program to search a specific item in a singly linked list and return true if the item is found otherwise return false.
Sample Solution:
Python Code:
Sample Output:
False True
Flowchart:
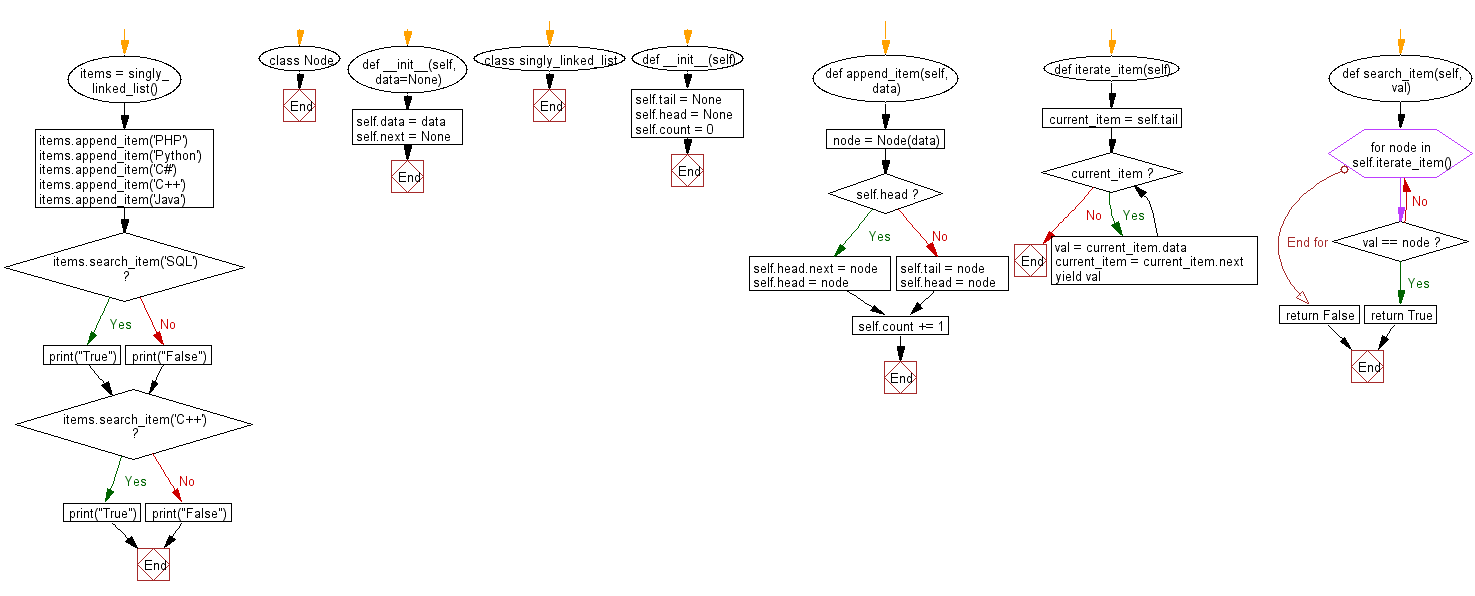
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to find the size of a singly linked list.
Next: Write a Python program to access a specific item in a singly linked list using index value.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics