Python Linked List: Create a singly linked list, append some items and iterate through the list
1. Singly Linked List Creation
Write a Python program to create a singly linked list, append some items and iterate through the list.
Sample Solution:
Python Code:
class Node:
# Singly linked node
def __init__(self, data=None):
self.data = data
self.next = None
class singly_linked_list:
def __init__(self):
# Createe an empty list
self.head = None
self.tail = None
self.count = 0
def iterate_item(self):
# Iterate the list.
current_item = self.head
while current_item:
val = current_item.data
current_item = current_item.next
yield val
def append_item(self, data):
#Append items on the list
node = Node(data)
if self.tail:
self.tail.next = node
self.tail = node
else:
self.head = node
self.tail = node
self.count += 1
items = singly_linked_list()
items.append_item('PHP')
items.append_item('Python')
items.append_item('C#')
items.append_item('C++')
items.append_item('Java')
for val in items.iterate_item():
print(val)
print("\nhead.data: ",items.head.data)
print("tail.data: ",items.tail.data)
Sample Output:
PHP Python C# C++ Java head.data: PHP tail.data: Java
Flowchart:
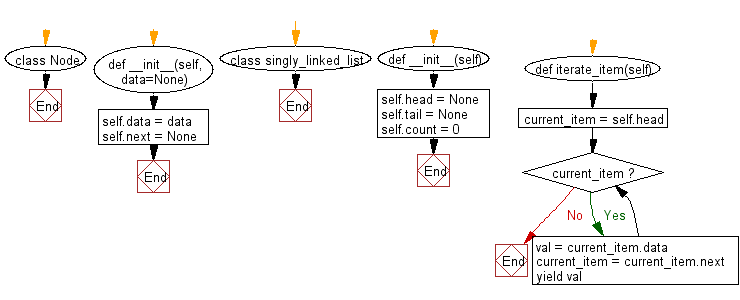
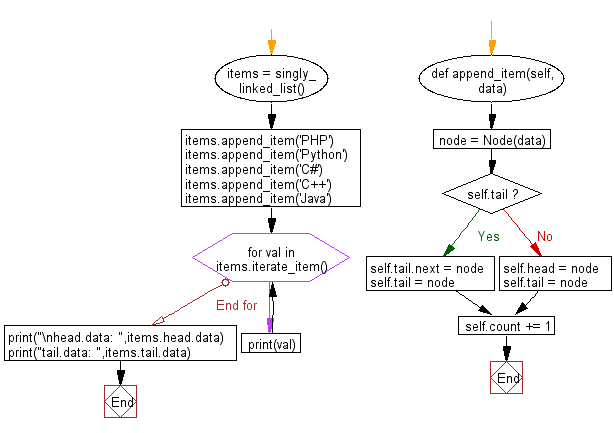
For more Practice: Solve these Related Problems:
- Write a Python program to create a singly linked list from a list of integers and then iterate over the list to display each element.
- Write a Python script to build a singly linked list by appending nodes, then use recursion to print all node values.
- Write a Python program to construct a singly linked list from user input, and iterate through it to calculate the sum of its elements.
- Write a Python function to create a singly linked list, append nodes with random values, and print each node’s value along with its memory address.
Go to:
Previous: Python Linked List Home.
Next: Write a Python program to find the size of a singly linked list.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.