Python Binary Search Tree: Find the closest value of a target in a given non-empty Binary Search Tree (BST) of unique values
2. Closest Value in BST
Write a Python program to find the closest value to a given target value in a given non-empty Binary Search Tree (BST) of unique values.
Sample Solution:
Python Code:
class TreeNode(object):
def __init__(self, x):
self.val = x
self.left = None
self.right = None
def closest_value(root, target):
a = root.val
kid = root.left if target < a else root.right
if not kid:
return a
b = closest_value(kid, target)
return min((a,b), key=lambda x: abs(target-x))
root = TreeNode(8)
root.left = TreeNode(5)
root.right = TreeNode(14)
root.left.left = TreeNode(4)
root.left.right = TreeNode(6)
root.left.right.left = TreeNode(8)
root.left.right.right = TreeNode(7)
root.right.right = TreeNode(24)
root.right.right.left = TreeNode(22)
result = closest_value(root, 19)
print(result)
Sample Output:
22
Flowchart:
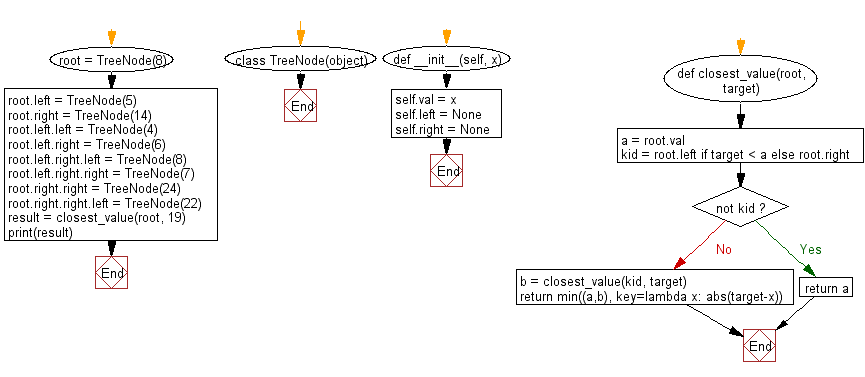
For more Practice: Solve these Related Problems:
- Write a Python program to search for the closest value to a target in a BST using an iterative approach and print the closest value found.
- Write a Python script to find the closest value to a given target in a BST and also return the difference between the target and the closest value.
- Write a Python program to implement a recursive function that navigates a BST and finds the value closest to a specified target, then test it on various target values.
- Write a Python function that, given a BST and a target, returns both the closest value and the path taken to reach that value.
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to create a Balanced Binary Search Tree (BST) using an array (given) elements where array elements are sorted in ascending order.
Next: Write a Python program to check whether a given a binary tree is a valid binary search tree (BST) or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.