Python: Create a deque from an existing iterable object
8. Create a Deque from an Existing Iterable Object
Write a Python program to create a deque from an existing iterable object.
Sample Solution:
Python Code:
# Import the collections module to use the deque data structure
import collections
# Create a tuple named 'even_nums' containing even numbers 2, 4, and 6
even_nums = (2, 4, 6)
# Print the original tuple
print("Original tuple:")
print(even_nums)
# Print the data type of the 'even_nums' tuple
print(type(even_nums))
# Create a deque 'even_nums_deque' from the 'even_nums' tuple
even_nums_deque = collections.deque(even_nums)
# Print the original deque
print("\nOriginal deque:")
print(even_nums_deque)
# Append the numbers 8, 10, 12 to the right of the deque
even_nums_deque.append(8)
even_nums_deque.append(10)
even_nums_deque.append(12)
# Add 2 to the left of the deque
even_nums_deque.appendleft(2)
# Print the updated deque
print("New deque from an existing iterable object:")
print(even_nums_deque)
# Print the data type of the 'even_nums_deque' deque
print(type(even_nums_deque))
Sample Output:
Original tuple: (2, 4, 6) <class 'tuple'> Original deque: deque([2, 4, 6]) New deque from an existing iterable object: deque([2, 2, 4, 6, 8, 10, 12]) <class 'collections.deque'>
Flowchart:
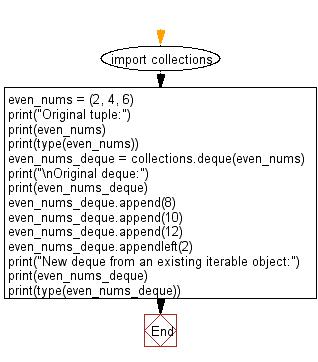
For more Practice: Solve these Related Problems:
- Write a Python program to convert a tuple into a deque and print the deque's type and contents.
- Write a Python program to create a deque from a string and iterate over its characters.
- Write a Python program to use collections.deque() to create a deque from a range of numbers and then convert it back to a list.
- Write a Python program to initialize a deque from an existing list and then perform basic operations like append and pop.
Python Code Editor:
Previous: Write a Python program to create a deque and append few elements to the left and right, then remove some elements from the left, right sides and reverse the deque.
Next: Write a Python program to add more number of elements to a deque object from an iterable object.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics