Python: Print subject name and marks in order of its first occurrence
Write a Python program that accepts the number of subjects, subject names and marks. Input the number of subjects and then the subject name and marks separated by a space on the next line. Print the subject name and marks in order of appearance.
Sample Solution:
Python Code:
# Import the collections and re modules
import collections, re
# Prompt the user to input the number of subjects
n = int(input("Number of subjects: "))
# Create an ordered dictionary to store subject names and their respective marks
item_order = collections.OrderedDict()
# Loop 'n' times to gather subject names and marks
for i in range(n):
# Prompt the user to input subject name and marks, split the input into subject_name and item_price
sub_marks_list = re.split(r'(\d+)$', input("Input Subject name and marks: ").strip())
subject_name = sub_marks_list[0]
item_price = int(sub_marks_list[1])
# Check if the subject_name is already in the item_order dictionary
if subject_name not in item_order:
# If not, add a new entry with the subject_name as the key and item_price as the value
item_order[subject_name] = item_price
else:
# If it already exists, update the item_order dictionary with the new item_price
item_order[subject_name] = item_order[subject_name] + item_price
# Iterate over the ordered dictionary and print subject names and their respective marks
for i in item_order:
print(i + str(item_order[i]))
Sample Output:
Powered by Number of subjects: 3 Input Subject name and marks: Bengali 58 Input Subject name and marks: English 62 Input Subject name and marks: Math 68 Bengali 58 English 62 Math 68
Flowchart:
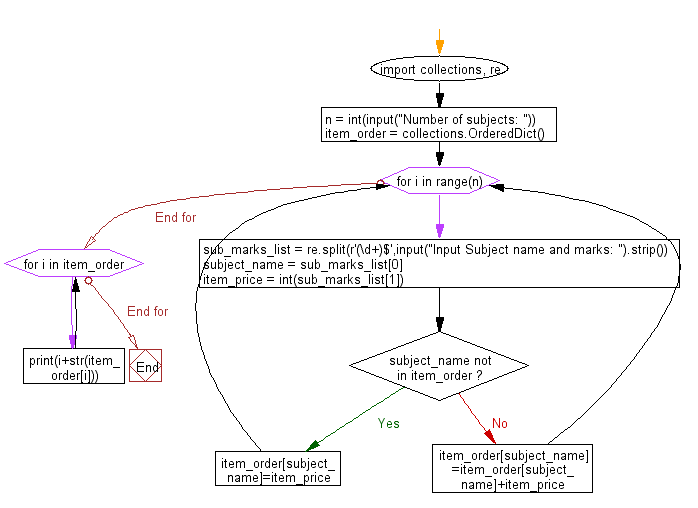
Python Code Editor:
Previous: Write a Python program that accept some words and count the number of distinct words. Print the number of distinct words and number of occurrences for each distinct word according to their appearance.
Next: Write a Python program to create a deque and append few elements to the left and right, then remove some elements from the left, right sides and reverse the deque.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics