Python: Accept some words and count the number of distinct words
Python Collections: Exercise-5 with Solution
Write a Python program that accepts some words and counts the number of distinct words. Print the number of distinct words and the number of occurrences of each distinct word according to their appearance.
Sample Solution:
Python Code:
# Import the Counter and OrderedDict classes from the collections module
from collections import Counter, OrderedDict
# Create a new class 'OrderedCounter' that inherits from both Counter and OrderedDict
class OrderedCounter(Counter, OrderedDict):
pass
# Create an empty list 'word_array' to store words
word_array = []
# Prompt the user to input the number of words
n = int(input("Input number of words: "))
# Prompt the user to input the words and store them in 'word_array'
print("Input the words: ")
for i in range(n):
word_array.append(input().strip())
# Create an instance of the 'OrderedCounter' class using 'word_array'
word_ctr = OrderedCounter(word_array)
# Print the number of unique words in 'word_ctr'
print(len(word_ctr))
# Iterate over the words in 'word_ctr' and print their counts, separated by space
for word in word_ctr:
print(word_ctr[word], end=' ')
Sample Output:
Input number of words: 5 Input the words: Red Green Blue Black White 5 1 1 1 1 1
Flowchart:
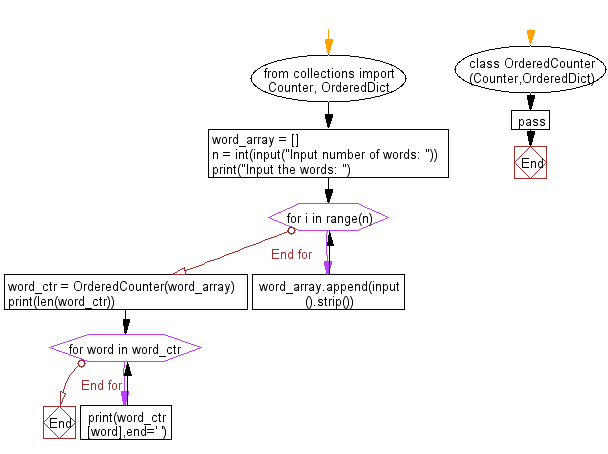
Python Code Editor:
Previous: Write a Python program to find the occurrences of 10 most common words in a given text.
Next: Write a Python program that accepts number of subjects, subject names and marks. Input number of subjects and then subject name, marks separated by a space in next line. Print subject name and marks in order of its first occurrence.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/collections/python-collections-exercise-5.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics