Python: Find the occurrences of 10 most common words in a given text
Python Collections: Exercise-4 with Solution
Write a Python program to find the occurrences of the 10 most common words in a given text.
Sample Solution:
Python Code:
# Import the Counter class from the collections module
from collections import Counter
# Import the re module for regular expressions
import re
# Define a multi-line text string containing information about Python
text = """The Python Software Foundation (PSF) is a 501(c)(3) non-profit
corporation that holds the intellectual property rights behind
the Python programming language. We manage the open source licensing
for Python version 2.1 and later and own and protect the trademarks
associated with Python. We also run the North American PyCon conference
annually, support other Python conferences around the world, and
fund Python related development with our grants program and by funding
special projects."""
# Use a regular expression to extract words from the text
words = re.findall('\w+', text)
# Use Counter to count the occurrences of each word in the text and find the 10 most common words
print(Counter(words).most_common(10))
Sample Output:
[('Python', 6), ('the', 6), ('and', 5), ('We', 2), ('with', 2), ('The', 1), ('Software', 1), ('Foundation', 1), ('PSF', 1), ('is', 1)]
Flowchart:
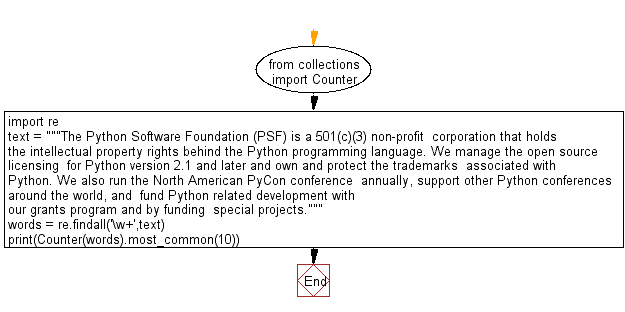
Python Code Editor:
Previous: Write a Python program to create a new deque with three items and iterate over the deque's elements.
Next: Write a Python program that accept some words and count the number of distinct words. Print the number of distinct words and number of occurrences for each distinct word according to their appearance.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/collections/python-collections-exercise-4.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics