Python: Sort the dictionary during the creation and print the members of the dictionary in reverse order
Write a Python program to create an instance of an OrderedDict using a given dictionary. Sort the dictionary during the creation and print the members of the dictionary in reverse order.
Sample Solution:
Python Code:
# Import the OrderedDict class from the collections module
from collections import OrderedDict
# Create a dictionary 'dict' with country names as keys and their international dialing codes as values
dict = {'Afghanistan': 93, 'Albania': 355, 'Algeria': 213, 'Andorra': 376, 'Angola': 244}
# Create a new OrderedDict 'new_dict' using the items of the original dictionary
new_dict = OrderedDict(dict.items())
# Loop through the keys in 'new_dict' and print each key and its corresponding value
for key in new_dict:
print(key, new_dict[key])
# Print a message to indicate the display of keys and values in reverse order
print("\nIn reverse order:")
# Loop through the keys in 'new_dict' in reverse order and print each key and its corresponding value
for key in reversed(new_dict):
print(key, new_dict[key])
Sample Output:
Afghanistan 93 Albania 355 Algeria 213 Andorra 376 Angola 244 In reverse order: Angola 244 Andorra 376 Algeria 213 Albania 355 Afghanistan 93
Flowchart:
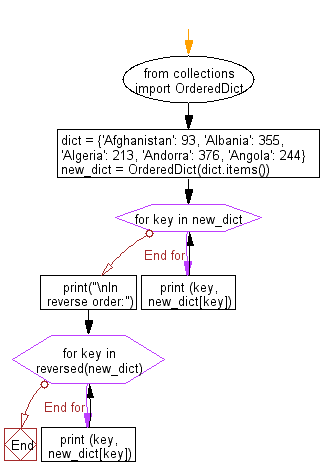
Python Code Editor:
Previous: Write a Python program to count the number of students of individual class.
Next: Write a Python program to group a sequence of key-value pairs into a dictionary of lists.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics