Python: Count the occurrence of each element of a given list
Python Collections: Exercise-30 with Solution
Write a Python program to count the occurrences of each element in a given list.
Sample Solution:
Python Code:
# Import the Counter class from the collections module
from collections import Counter
# Create a list 'colors' with strings representing colors
colors = ['Green', 'Red', 'Blue', 'Red', 'Orange', 'Black', 'Black', 'White', 'Orange']
# Print a message to indicate the display of the original list
print("Original List:")
# Print the content of the 'colors' list
print(colors)
# Print a message to indicate the display of the count of occurrences of each element in the list
print("Count the occurrence of each element of the said list:")
# Use Counter to count the occurrences of elements in 'colors' and store the result in 'result'
result = Counter(colors)
# Print the result
print(result)
# Create a list 'nums' with integer values
nums = [3, 5, 0, 3, 9, 5, 8, 0, 3, 8, 5, 8, 3, 5, 8, 1, 0, 2]
# Print a message to indicate the display of the original list
print("\nOriginal List:")
# Print the content of the 'nums' list
print(nums)
# Print a message to indicate the display of the count of occurrences of each element in the list
print("Count the occurrence of each element of the said list:")
# Use Counter to count the occurrences of elements in 'nums' and store the result in 'result'
result = Counter(nums)
# Print the result
print(result)
Sample Output:
Original List:
['Green', 'Red', 'Blue', 'Red', 'Orange', 'Black', 'Black', 'White', 'Orange'] Count the occurrence of each element of the said list: Counter({'Red': 2, 'Orange': 2, 'Black': 2, 'Green': 1, 'Blue': 1, 'White': 1}) Original List:
[3, 5, 0, 3, 9, 5, 8, 0, 3, 8, 5, 8, 3, 5, 8, 1, 0, 2] Count the occurrence of each element of the said list: Counter({3: 4, 5: 4, 8: 4, 0: 3, 9: 1, 1: 1, 2: 1})
Flowchart:
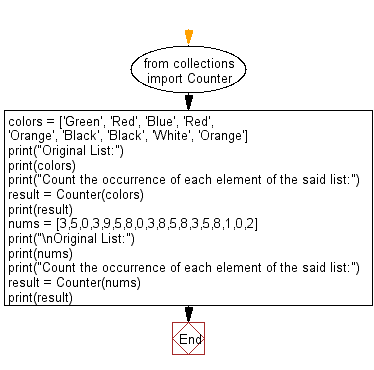
Python Code Editor:
Previous: Write a Python program to get the frequency of the elements in a given list of lists. Use collections module.
Next: Write a Python program to count the most common words in a dictionary.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/collections/python-collections-exercise-30.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics