Python: Break a list of integers into sets of a given positive number
19. Break a List of Integers into Sets of a Given Positive Number
Write a Python program to break a given list of integers into sets of a given positive number. Return true or false.
Sample Solution:
Python Code:
# Import the collections module and alias it as 'clt'
import collections as clt
# Define a function 'check_break_list' that checks if a list can be divided into consecutive sublists
def check_break_list(nums, n):
# Create a Counter object 'coll_data' to count the occurrences of elements in 'nums'
coll_data = clt.Counter(nums)
# Loop through the sorted keys of 'coll_data'
for x in sorted(coll_data.keys()):
# Iterate from 1 to 'n-1'
for index in range(1, n):
# Update 'coll_data' to account for the division of elements into sublists
coll_data[x + index] = coll_data[x + index] - coll_data[x]
# If the count becomes negative, it's not possible to divide the list
if coll_data[x + index] < 0:
return False
# If the loop completes, it's possible to divide the list into consecutive sublists
return True
# Create a list 'nums' and an integer 'n'
nums = [1, 2, 3, 4, 5, 6, 7, 8]
n = 4
# Print a message to indicate the display of the original list and the number for division
print("Original list:", nums)
print("Number to divide the said list:", n)
# Call the 'check_break_list' function and print the result
print(check_break_list(nums, n))
# Create another list 'nums' and a different integer 'n'
nums = [1, 2, 3, 4, 5, 6, 7, 8]
n = 3
# Print a message to indicate the display of the original list and the number for division
print("\nOriginal list:", nums)
print("Number to divide the said list:", n)
# Call the 'check_break_list' function and print the result
print(check_break_list(nums, n))
Sample Output:
Original list: [1, 2, 3, 4, 5, 6, 7, 8] Number to devide the said list: 4 True Original list: [1, 2, 3, 4, 5, 6, 7, 8] Number to devide the said list: 3 False
Flowchart:
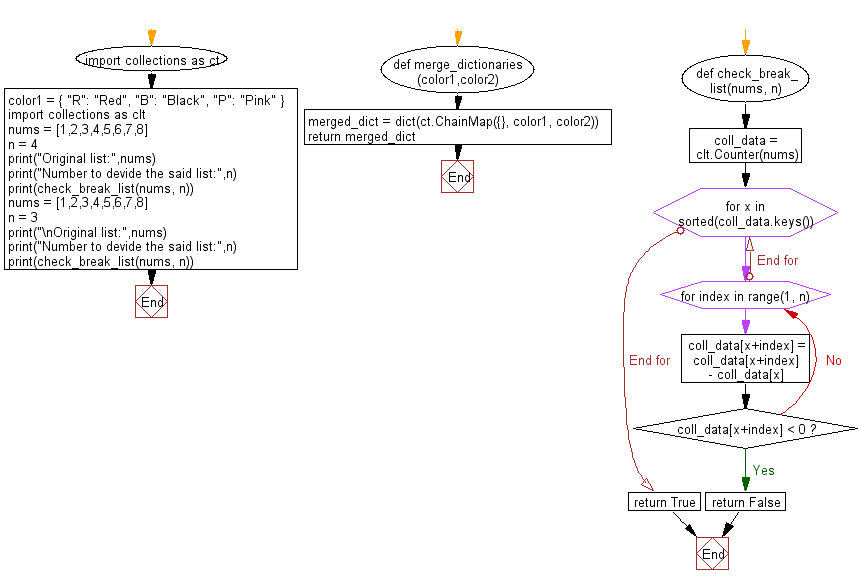
For more Practice: Solve these Related Problems:
- Write a Python program to divide a list into subsets of a specified size and return True if the list can be perfectly partitioned.
- Write a Python program to check if a list’s length is divisible by a given number and then break it into equal-sized sets.
- Write a Python program to implement a function that partitions a list into chunks of a given size and returns a boolean indicating if the partition was perfect.
- Write a Python program to compare the length of a list with a divisor and output the list of sublists if it divides evenly.
Go to:
Previous: Write a Python program to merge more than one dictionary in a single expression.
Next: Write a Python program to find the item with maximum frequency in a given list.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.