Python: Merge more than one dictionary in a single expression
Python Collections: Exercise-18 with Solution
Write a Python program to merge more than one dictionary into a single expression.
Sample Solution:
Python Code:
# Import the collections module and alias it as 'ct'
import collections as ct
# Define a function 'merge_dictionaries' that takes two dictionaries as input and merges them using ChainMap
def merge_dictionaries(color1, color2):
# Create a merged dictionary using ChainMap by chaining the provided dictionaries
merged_dict = dict(ct.ChainMap({}, color1, color2))
return merged_dict
# Create two dictionaries 'color1' and 'color2'
color1 = { "R": "Red", "B": "Black", "P": "Pink" }
color2 = { "G": "Green", "W": "White" }
# Print a message to indicate the display of the original dictionaries
print("Original dictionaries:")
# Print the content of 'color1' and 'color2'
print(color1, ' ', color2)
# Print a message to indicate the display of the merged dictionary
print("\nMerged dictionary:")
# Call the 'merge_dictionaries' function with 'color1' and 'color2' and print the result
print(merge_dictionaries(color1, color2))
# Define a function 'merge_dictionaries' that takes three dictionaries as input and merges them using ChainMap
def merge_dictionaries(color1, color2, color3):
# Create a merged dictionary using ChainMap by chaining the provided dictionaries
merged_dict = dict(ct.ChainMap({}, color1, color2, color3))
return merged_dict
# Create three dictionaries 'color1', 'color2', and 'color3'
color1 = { "R": "Red", "B": "Black", "P": "Pink" }
color2 = { "G": "Green", "W": "White" }
color3 = { "O": "Orange", "W": "White", "B": "Black" }
# Print a message to indicate the display of the original dictionaries
print("\nOriginal dictionaries:")
# Print the content of 'color1', 'color2', and 'color3'
print(color1, ' ', color2, color3)
# Print a message to indicate the display of the merged dictionary
print("\nMerged dictionary:")
# Call the 'merge_dictionaries' function with 'color1', 'color2', and 'color3' and print the result
# Duplicate colors have been automatically removed due to ChainMap
print(merge_dictionaries(color1, color2, color3))
Sample Output:
Original dictionaries: {'R': 'Red', 'B': 'Black', 'P': 'Pink'} {'G': 'Green', 'W': 'White'} Merged dictionary: {'B': 'Black', 'R': 'Red', 'P': 'Pink', 'G': 'Green', 'W': 'White'} Original dictionaries: {'R': 'Red', 'B': 'Black', 'P': 'Pink'} {'G': 'Green', 'W': 'White'} {'O': 'Orange', 'W': 'White', 'B': 'Black'} Merged dictionary: {'B': 'Black', 'R': 'Red', 'P': 'Pink', 'G': 'Green', 'W': 'White', 'O': 'Orange'}
Flowchart:
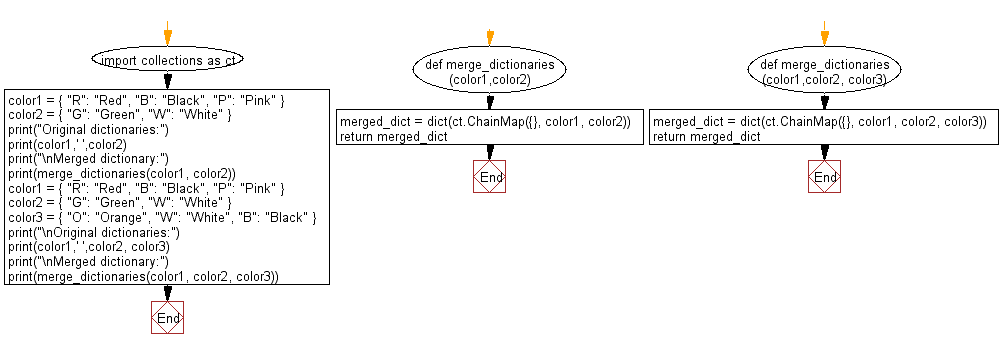
Python Code Editor:
Previous: Write a Python program to find the majority element from a given array of size n using Collections module.
Next: Write a Python program to break a given list of integers into sets of a given positive number. Return true or false.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/collections/python-collections-exercise-18.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics