Python: Find the second lowest total marks of any student(s) from the given names and total marks of each student using lists and Lambda
Write a Python program to find the second lowest total marks of any student(s) from the given names and marks of each student using lists and lambda. Input number of students, names and grades of each student.
Sample Solution:
Python Code:
# Create an empty list 'students' to store student names and their scores
students = []
# Create an empty list 'sec_name' (currently unused)
sec_name = []
# Initialize the variable 'second_low' to 0
second_low = 0
# Prompt the user to input the number of students
n = int(input("Input number of students: "))
# Loop to input names and scores for 'n' students
for _ in range(n):
# Prompt the user to input the student's name
s_name = input("Name: ")
# Prompt the user to input the student's total marks (score)
score = float(input("Total marks: "))
# Append a list containing the student's name and score to the 'students' list
students.append([s_name, score])
# Print a message to display the names and marks of all students
print("\nNames and Marks of all students:")
# Print the content of the 'students' list
print(students)
# Sort the 'students' list based on the scores in ascending order
order = sorted(students, key=lambda x: int(x[1]))
# Loop to find the second lowest score
for i in range(n):
if order[i][1] != order[0][1]:
second_low = order[i][1]
break
# Print a message to display the second lowest score
print("\nSecond lowest Marks: ", second_low)
# Create a list 'sec_student_name' to store names of students with the second lowest score
sec_student_name = [x[0] for x in order if x[1] == second_low]
# Sort the names in 'sec_student_name' in alphabetical order
sec_student_name.sort()
# Print a message to display the names of students with the second lowest score
print("\nNames:")
# Loop to print the names of these students
for s_name in sec_student_name:
print(s_name)
Sample Output:
Input number of students: 3 Name: Avik Das Total marks: 89 Name: ayan Roy Total marks: 75 Name: Sayan Dutta Total marks: 93 Names and Marks of all students: [['Avik Das ', 89.0], ['ayan Roy', 75.0], ['Sayan Dutta', 93.0]] Second lowest Marks: 89.0 Names: Avik Das
Flowchart:
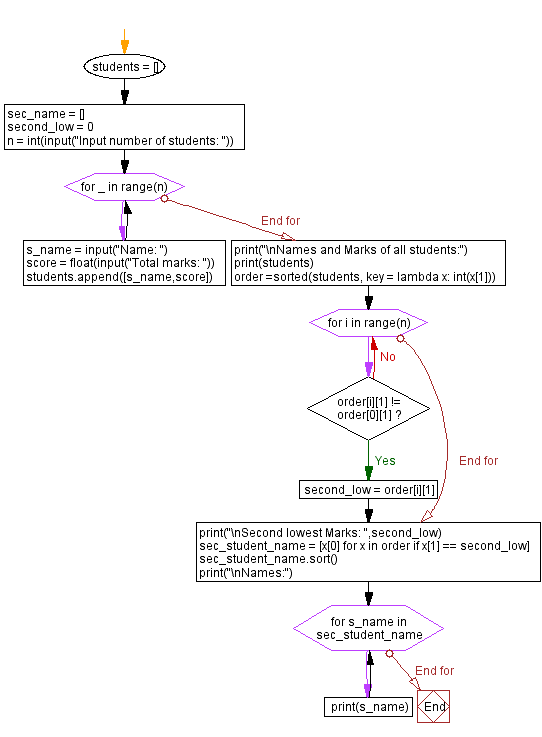
Python Code Editor:
Previous: Write a Python program to find the most common element of a given list.
Next: Write a Python program to find the majority element from a given array of size n using Collections module.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics