Python: Find the most common element of a given list
15. Find the Most Common Element in a List
Write a Python program to find the most common element in a given list.
Sample Solution:
Python Code:
# Import the Counter class from the collections module
from collections import Counter
# Create a list 'language' containing programming language names
language = ['PHP', 'PHP', 'Python', 'PHP', 'Python', 'JS', 'Python', 'Python', 'PHP', 'Python']
# Print a message to indicate the display of the original list
print("Original list:")
# Print the content of the 'language' list
print(language)
# Create a Counter object 'cnt' to count the occurrences of each language in the list
cnt = Counter(language)
# Print a message to indicate the display of the most common element in the list
print("\nMost common element of the said list:")
# Find and print the most common element (programming language) in the list
# Note: In this case, it returns the first most common element
print(cnt.most_common(1)[0][0])
Sample Output:
Original list: ['PHP', 'PHP', 'Python', 'PHP', 'Python', 'JS', 'Python', 'Python', 'PHP', 'Python'] Most common element of the said list: Python
Flowchart:
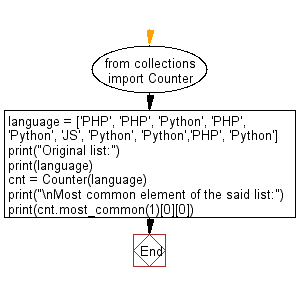
For more Practice: Solve these Related Problems:
- Write a Python program to identify the most frequent element in a list using collections.Counter.
- Write a Python program to iterate through a list and determine which element appears the most, then return that element and its count.
- Write a Python program to use a dictionary to count occurrences and then print the element with the highest frequency.
- Write a Python program to implement a function that returns the most common element, handling ties by returning the smallest element.
Go to:
Previous: Write a Python program to rotate a Deque Object specified number (negative) of times.
Next: Write a Python program to find the second lowest total marks of any student(s) from the given names and marks of each student using lists and lambda. Input number of students, names and grades of each student.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.