Python: Find the three elements that sum to zero from a set of n real numbers
6. Find Three Elements that Sum to Zero
Write a Python class to find the three elements that sum to zero from a set (array) of n real numbers.
Sample Solution:
Python Code:
class py_solution:
def threeSum(self, nums):
nums, result, i = sorted(nums), [], 0
while i < len(nums) - 2:
j, k = i + 1, len(nums) - 1
while j < k:
if nums[i] + nums[j] + nums[k] < 0:
j += 1
elif nums[i] + nums[j] + nums[k] > 0:
k -= 1
else:
result.append([nums[i], nums[j], nums[k]])
j, k = j + 1, k - 1
while j < k and nums[j] == nums[j - 1]:
j += 1
while j < k and nums[k] == nums[k + 1]:
k -= 1
i += 1
while i < len(nums) - 2 and nums[i] == nums[i - 1]:
i += 1
return result
print(py_solution().threeSum([-25, -10, -7, -3, 2, 4, 8, 10]))
Sample Output:
[[-10, 2, 8], [-7, -3, 10]]
Pictorial Presentation:
Flowchart:
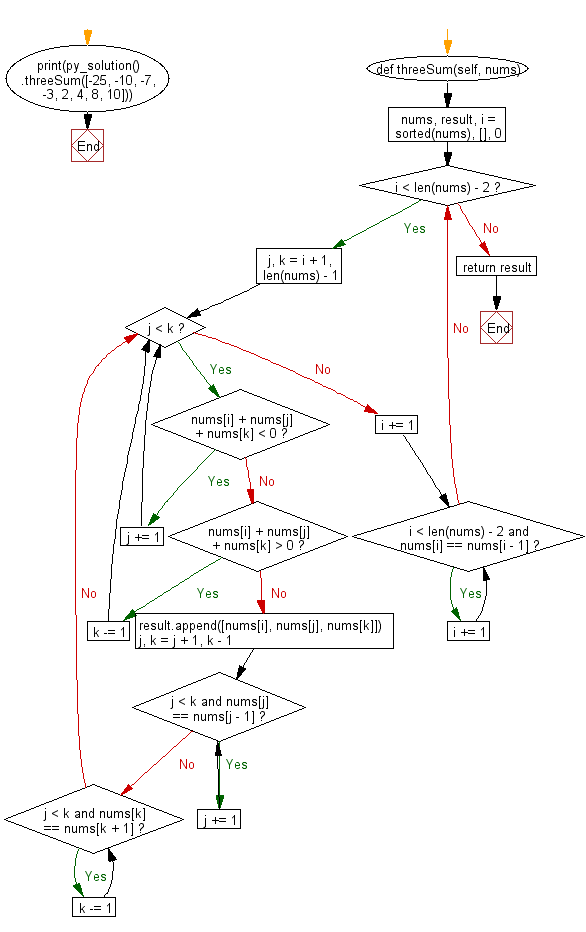
For more Practice: Solve these Related Problems:
- Write a Python class that uses a brute-force approach with three nested loops to find all triplets in an array that sum to zero.
- Write a Python class that sorts the input array and then uses a two-pointer technique for each element to find zero-sum triplets.
- Write a Python class that implements a function using recursion to generate all combinations of three numbers and filter those that sum to zero.
- Write a Python class that leverages sets to avoid duplicate triplets when finding all three-element combinations that sum to zero.
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python class to find a pair of elements (indices of the two numbers) from a given array whose sum equals a specific target number.
Next: Write a Python class to implement pow(x, n).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.