Python: Print all the attributes and their values of instances
12. Create a Class Named Student, Instantiate Two Instances, and Print Their Attributes
Write a Python class named Student with two instances student1, student2 and assign values to the instances' attributes. Print all the attributes of the student1, student2 instances with their values in the given format.
Input values of the instances:
student_1:
student_id = "V12"
student_name = "Ernesto Mendez"
student_2:
student_id = "V12"
marks_language = 85
marks_science = 93
marks_math = 95
Expected Output:
student_id -> V12
student_name -> Ernesto Mendez
student_id -> V12
marks_language -> 85
marks_science -> 93
marks_math -> 95
Sample Solution:
Python Code:
class Student:
school = 'Forward Thinking'
address = '2626/Z Overlook Drive, COLUMBUS'
student1 = Student()
student2 = Student()
student1.student_id = "V12"
student1.student_name = "Ernesto Mendez"
student2.student_id = "V12"
student2.marks_language = 85
student2.marks_science = 93
student2.marks_math = 95
students = [student1, student2]
for student in students:
print('\n')
for attr in student.__dict__:
print(f'{attr} -> {getattr(student, attr)}')
Sample Output:
student_id -> V12 student_name -> Ernesto Mendez student_id -> V12 marks_language -> 85 marks_science -> 93 marks_math -> 95
Flowchart:
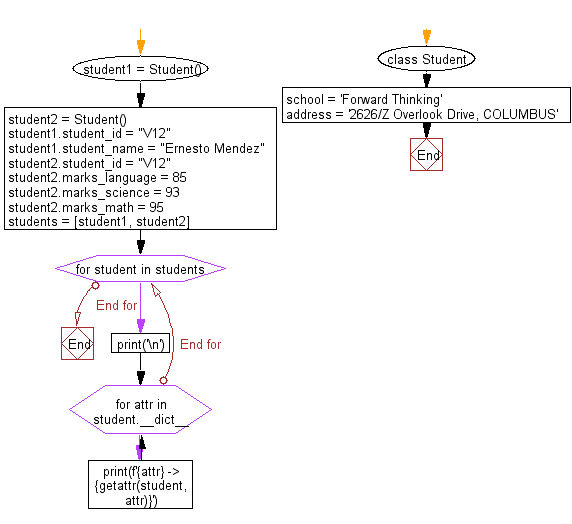
For more Practice: Solve these Related Problems:
- Write a Python program to define a Student class, create two instances with different attribute values, and print each instance's __dict__.
- Write a Python program to compare the attributes of two Student instances and display them in a formatted output.
- Write a Python program to implement a function that takes two Student objects and prints their attributes side by side.
- Write a Python program to use a loop to iterate over a list of Student instances and print each student's details.
Go to:
Previous: Write a Python class named Student with two attributes student_id, student_name. Add a new attribute student_class. Create a function to display the entire attribute and their values in Student class.
Next: Write a Python class to convert an integer to a roman numeral.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.