Python Challenges: Each element at index i of an array is the product of all the numbers of a given array except the one at i
Write a Python program to create a new array such that each element at index i of the new array is the product of all the numbers of a given array of integers except the one at i.
Input: [10, 20, 30, 40, 50]
Output: [1200000, 600000, 400000, 300000, 240000]
Input: [1, 2, 0, 4]
Output: [0, 0, 8, 0]
Sample Solution:
Python Code:
def product(nums):
new_nums = []
for i in nums:
nums_product = 1
for j in nums:
if j != i:
nums_product = nums_product * j
new_nums.append(nums_product)
return new_nums
print(product([10, 20, 30, 40, 50]))
print(product([1, 2, 0, 4]))
print(product([1, 2, 3, -4]))
Sample Output:
[1200000, 600000, 400000, 300000, 240000] [0, 0, 8, 0] [-24, -12, -8, 6]
Flowchart:
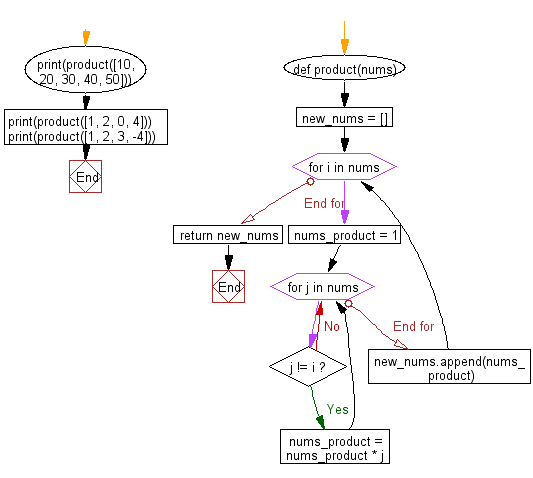
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to find equilibrium index from a given array. If there is no equilibrium index return 1.
Next: Write a Python program to compute the person's itinerary. If no such itinerary exists, return null. If there are multiple possible itineraries, return the lexicographically smallest one. All flights must be used in the itinerary.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.