Python Challenges: Compute the edit distance between two given strings
From Wikipedia, the free encyclopedia
In computational linguistics and computer science, edit distance is a way of quantifying how dissimilar two strings (e.g., words) are to one another by counting the minimum number of operations required to transform one string into the other. Edit distances find applications in natural language processing, where automatic spelling correction can determine candidate corrections for a misspelled word by selecting words from a dictionary that have a low distance to the word in question. In bioinformatics, it can be used to quantify the similarity of DNA sequences, which can be viewed as strings of the letters A, C, G and T.
This problem was asked by Google.
The edit distance between two strings refers to the minimum number of
character insertions, deletions, and substitutions required to change one
string to the other. For example, the edit distance between "kitten" and
"sitting" is three: substitute the "k" for "s", substitute the "e" for "i",
and append a "g".
Write a Python program to compute the edit distance between two given strings.
Sample Solution:
Python Code:
def edit_distance(string1, string2):
"""Ref: https://bit.ly/2Pf4a6Z"""
if len(string1) > len(string2):
difference = len(string1) - len(string2)
string1[:difference]
elif len(string2) > len(string1):
difference = len(string2) - len(string1)
string2[:difference]
else:
difference = 0
for i in range(len(string1)):
if string1[i] != string2[i]:
difference += 1
return difference
print(edit_distance("kitten", "sitting")) #3
print(edit_distance("medium", "median")) #2
Sample Output:
3 2
Flowchart:
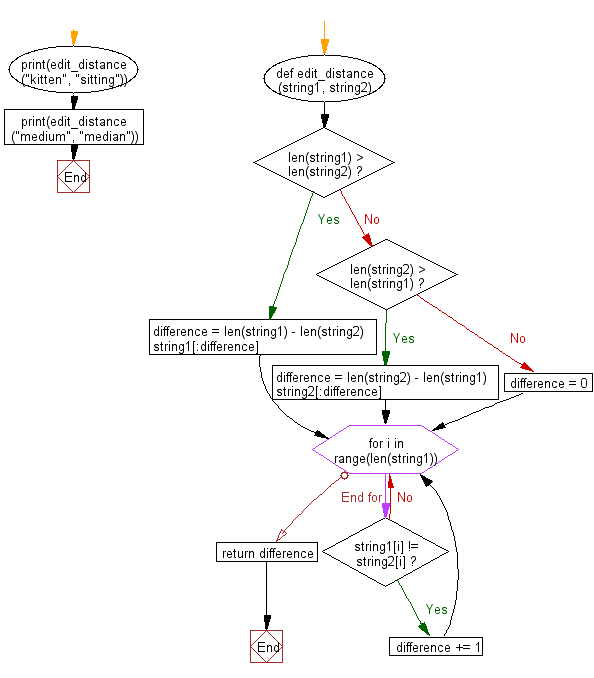
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program that takes a string of numbers and letters and return string which consists of letters.
Next: Write a Python program to find the largest palindrome made from the product of two 4-digit numbers
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.