Python Challenges: Find the index of the first term in the Fibonacci sequence to contain 500 digits
Write a Python program to find the index of the first term in the Fibonacci sequence to contain 500 digits.
In mathematics, the Fibonacci numbers, commonly denoted Fn form a sequence, called the Fibonacci sequence, such that each number is the sum of the two preceding ones, starting from 0 and 1
The Fibonacci sequence is defined by the recurrence relation:
Fn = Fn−1 + Fn−2, where F1 = 1 and F2 = 1.
Hence the first 12 terms will be:
F1 = 1
F2 = 1
F3 = 2
F4 = 3
F5 = 5
F6 = 8
F7 = 13
F8 = 21
F9 = 34
F10 = 55
F11 = 89
F12 = 144
The 12th term, F12, is the first term to contain three digits.
Sample Solution:
Python Code:
import itertools
def Fibonacci_sequence(n):
DIGITS = n
prev_num = 1
cur_num = 0
for i in itertools.count():
# At this point, prev = fibonacci(i - 1) and cur = fibonacci(i)
if len(str(cur_num)) > DIGITS:
raise RuntimeError("Not found")
elif len(str(cur_num)) == DIGITS:
return str(i)
prev_num, cur_num = cur_num, prev_num + cur_num
print(Fibonacci_sequence(500))
Sample Output:
2390
Flowchart:
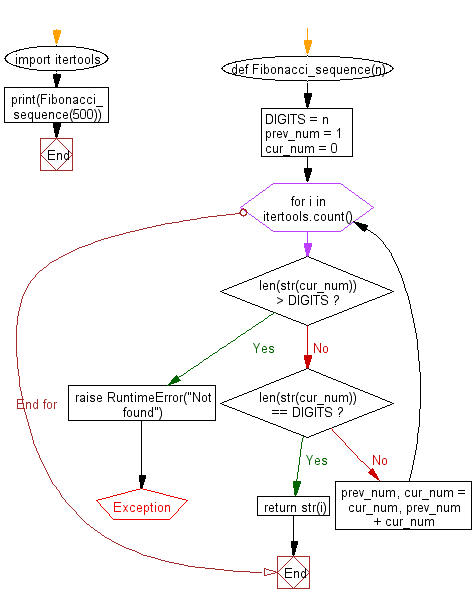
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to find the millionth lexicographic permutation of the digits 0, 1, 2, 3, 4, 5, 6, 7, 8 and 9.
Next: Write a Python program that takes a string and encode it that the amount of symbols would be represented by integer and the symbol.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics