Python Challenges: Find the largest prime factor of a given number
Write a Python program to find the largest prime factor of a given number.
The prime factors of 330 are 2, 3, 5 and 11. Therefore 11 is the largest prime factor of 330.
Sample Example: 330 = 2 × 3 × 5 × 11
Sample Solution:
Python Code:
def Largest_Prime_Factor(n):
return next(n // i for i in range(1, n) if n % i == 0 and is_prime(n // i))
def is_prime(m):
return all(m % i for i in range(2, m - 1))
print(Largest_Prime_Factor(200))
print(Largest_Prime_Factor(330))
print(Largest_Prime_Factor(243423423330))
Sample Output:
5 11 1114117
Flowchart:
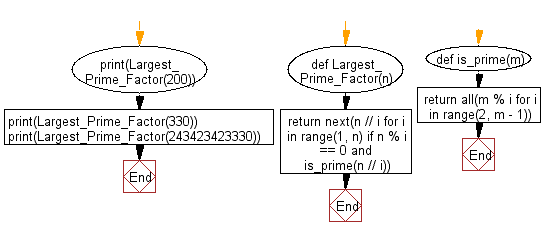
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to compute the sum of the even-valued terms in the Fibonacci sequence whose values do not exceed one million.
Next: Write a Python program to find the largest palindrome made from the product of two 4-digit numbers
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.