Python Challenges: Check whether a given number is an ugly number
Write a Python program to check whether a given number is an ugly number.
Ugly numbers are positive numbers whose only prime factors are 2, 3 or 5. The sequence 1, 2, 3, 4, 5, 6, 8, 9, 10, 12, ...
shows the first 10 ugly numbers.
Note: 1 is typically treated as an ugly number.
Explanation:
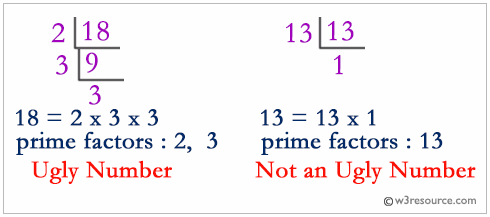
Sample Solution:
Python Code:
def is_ugly(num):
if num == 0:
return False
for i in [2, 3, 5]:
while num % i == 0:
num /= i
return num == 1
print(is_ugly(12))
print(is_ugly(13))
Sample Output:
True False
Flowchart:
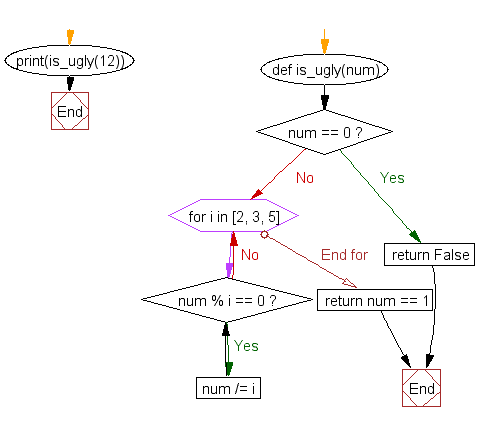
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program whereyou take any positive integer n, if n is even, divide it by 2 to get n / 2. If n is odd, multiply it by 3 and add 1 to obtain 3n + 1. Repeat the process until you reach 1.
Next: Write a Python program to get the Hamming numbers upto a given numbers also check whether a given number is an Hamming number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.