Python Challenges: Reverse the digits of an integer
Write a Python program to reverse the digits of an integer.
Explanation:
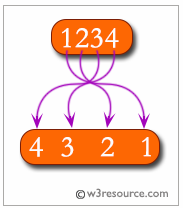
Sample Example:
Input : 234
Input : -234
Output: 432
Output : -432
Sample Solution-1:
Python Code:
def reverse_integer(x):
sign = -1 if x < 0 else 1
x *= sign
# Remove leading zero in the reversed integer
while x:
if x % 10 == 0:
x /= 10
else:
break
# string manipulation
x = str(x)
lst = list(x) # list('234') returns ['2', '3', '4']
lst.reverse()
x = "".join(lst)
x = int(x)
return sign*x
print(reverse_integer(234))
print(reverse_integer(-234))
Sample Output:
432 -432
Flowchart:
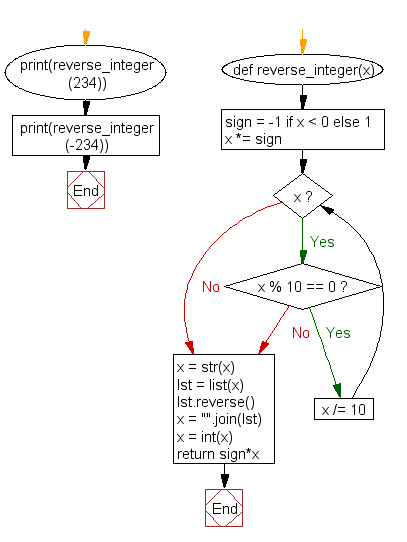
Sample Solution-2:
Reverses a number:
- Use str() to convert the number to a string, slice notation to reverse it and str.replace() to remove the sign.
- Use float() to convert the result to a number and math.copysign() to copy the original sign.
Python Code:
from math import copysign
def reverse_number(n):
return copysign(float(str(n)[::-1].replace('-', '')), n)
print(reverse_number(873))
print(reverse_number(-900))
print(reverse_number(234.6))
print(reverse_number(-15.78))
Sample Output:
378.0 -9.0 6.432 -87.51
Flowchart:
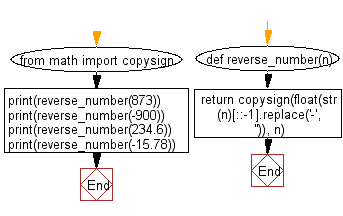
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to find whether it contains an additive sequence or not.
Next: Write a Python program to reverse the bits of an integer (32 bits unsigned).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics