Python Challenges: Find two elements once in a list where every element appears exactly twice in the list
Write a Python program to find two elements once in a list where every element appears exactly twice in the list.
Explanation :
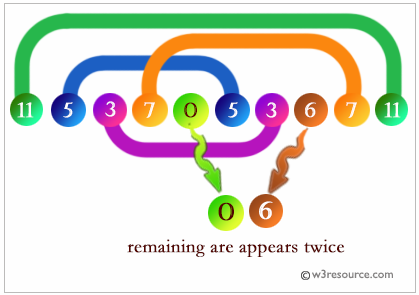
Sample Solution:
Python Code:
import functools
import operator
def two_numbers(arr):
x_xor_y = functools.reduce(operator.xor, arr)
bit = x_xor_y & -x_xor_y
result = [0, 0]
for i in arr:
result[bool(i & bit)] ^= i
return result
print(two_numbers([1, 2, 1, 3, 2, 5]))
print(two_numbers([11, 5, 3, 7, 0, 5, 3, 6, 7, 11]))
Sample Output:
[5, 3] [0, 6]
Flowchart:
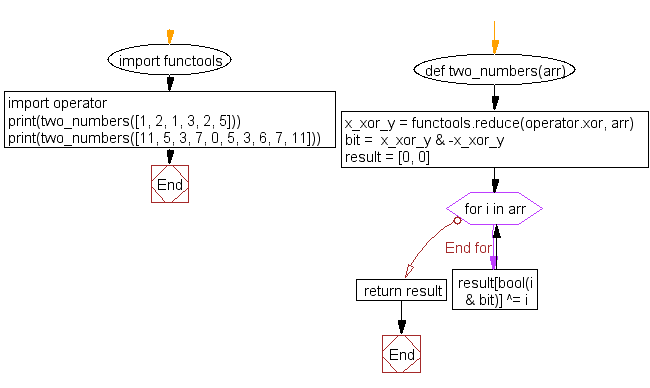
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to find the single element appears once in a list where every element appears four times except for one.
Next: Write a Python program to add the digits of a positive integer repeatedly until the result has a single digit.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.