Python Challenges: Find three numbers from an array such that the sum of three numbers equal to a given number
Write a Python program to find three numbers from an array such that the sum of three numbers equal to a given number.
Sample Solution:
Python Code:
def four_Sum(nums, target):
nums.sort()
result = []
for i in range(len(nums) - 3):
if i and nums[i] == nums[i - 1]:
continue
for j in range(i + 1, len(nums) - 2):
if j != i + 1 and nums[j] == nums[j - 1]:
continue
sum = target - nums[i] - nums[j]
left, right = j + 1, len(nums) - 1
while left < right:
if nums[left] + nums[right] == sum:
result.append([nums[i], nums[j], nums[left], nums[right]])
right -= 1
left += 1
while left < right and nums[left] == nums[left - 1]:
left += 1
while left < right and nums[right] == nums[right + 1]:
right -= 1
elif nums[left] + nums[right] > sum:
right -= 1
else:
left += 1
return result
print(four_Sum([1, 0, -1, 0, -2, 2], 0))
Sample Output:
[[-2, -1, 1, 2], [-2, 0, 0, 2], [-1, 0, 0, 1]]
Flowchart:
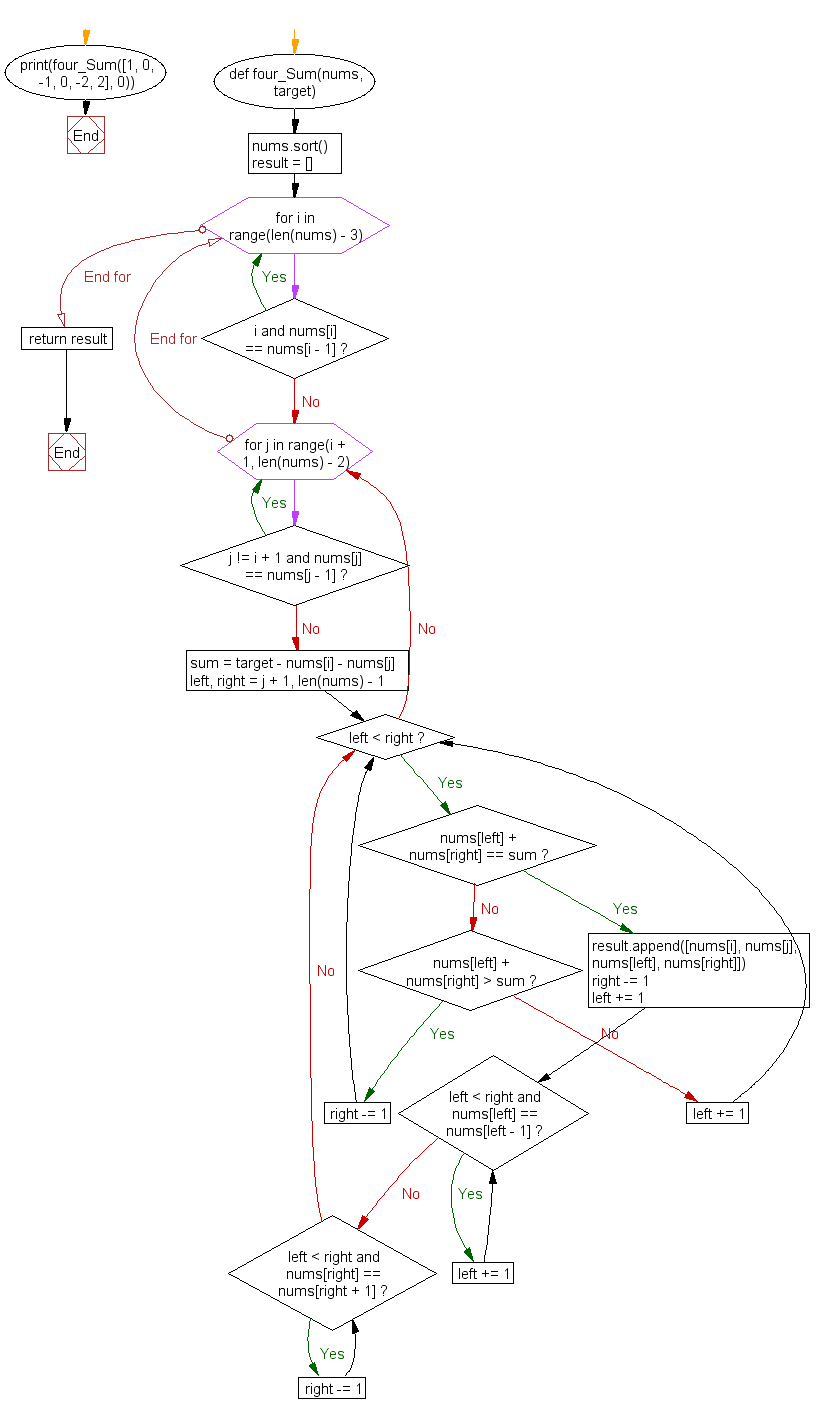
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to find three numbers from an array such that the sum of three numbers equal to zero.
Next: Write a Python program to compute and return the square root of a given 'integer'.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.