Python Bisect: Find the index position of the largest value smaller than a given number in a sorted list using Binary Search
5. Largest Smaller Value Index
Write a Python program to find the index position of the largest value smaller than a given number in a sorted list using Binary Search (bisect).
Sample Solution:
Python Code:
from bisect import bisect_left
def Binary_Search(l, x):
i = bisect_left(l, x)
if i:
return (i-1)
else:
return -1
nums = [1, 2, 3, 4, 8, 8, 10, 12]
x = 5
num_position = Binary_Search(nums, x)
if num_position == -1:
print("Not found..!")
else:
print("Largest value smaller than ", x, " is at index ", num_position )
Sample Output:
Largest value smaller than 5 is at index 3
Flowchart:
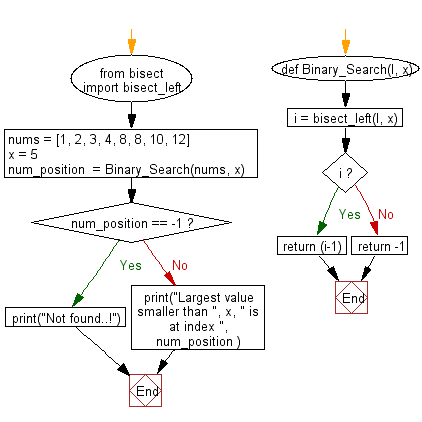
For more Practice: Solve these Related Problems:
- Write a Python program to find the index of the largest number smaller than a given target in a sorted list using bisect_left.
- Write a Python script to implement a function that returns the index of the element immediately preceding where a target would be inserted in a sorted list.
- Write a Python program to use binary search techniques to determine the index of the greatest element less than a specified value and print that index.
- Write a Python function that finds and returns the index of the largest value that is smaller than the target in a sorted list, including edge case handling when no such element exists.
Go to:
Next: Write a Python program to find the index position of the last occurrence of a given number in a sorted list using Binary Search (bisect).
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.