Python Bisect: Insert items into a list in sorted order
Write a Python program to insert items into a list in sorted order.
Sample Solution:
Python Code:
import bisect
# Sample list
my_list = [25, 45, 36, 47, 69, 48, 68, 78, 14, 36]
print("Original List:")
print(my_list)
sorted_list = []
for i in my_list:
position = bisect.bisect(sorted_list, i)
bisect.insort(sorted_list, i)
print("Sorted List:")
print(sorted_list)
Sample Output:
Original List: [25, 45, 36, 47, 69, 48, 68, 78, 14, 36] Sorted List: [14, 25, 36, 36, 45, 47, 48, 68, 69, 78]
Flowchart:
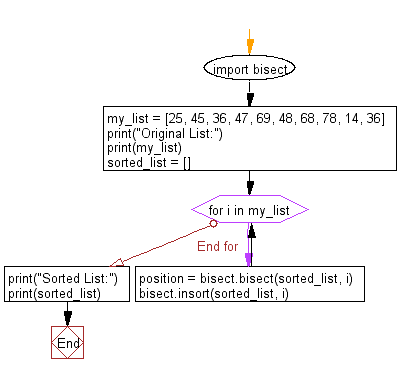
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to locate the right insertion point for a specified value in sorted order.
Next: Write a Python program to find the first occurrence of a given number in a sorted list using Binary Search (bisect).What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics