Python: Check every even index contains an even number and odd index contains odd number of a given list
Even-Odd Index Checker
Write a Python program that checks whether every even index contains an even number and every odd index contains an odd number of a given list.
Sample Solution:
Python Code:
# Define a function named odd_even_position that takes a list of numbers (nums) as an argument.
def odd_even_position(nums):
# Use the all() function along with a generator expression to check if every even index contains an even number
# and every odd index contains an odd number in the given list.
return all(nums[i] % 2 == i % 2 for i in range(len(nums)))
# Test the function with different lists of numbers and print the results.
# Test case 1
nums = [2, 1, 4, 3, 6, 7, 6, 3]
# Print the original list of numbers.
print("Original list of numbers:", nums)
# Print whether every even index contains an even number and every odd index contains an odd number.
print("Check whether every even index contains an even number and every \nodd index contains odd number of a given list:")
print(odd_even_position(nums))
# Test case 2
nums = [2, 1, 4, 3, 6, 7, 6, 4]
# Print the original list of numbers.
print("\nOriginal list of numbers:", nums)
# Print whether every even index contains an even number and every odd index contains an odd number.
print("Check whether every even index contains an even number and every \nodd index contains odd number of a given list:")
print(odd_even_position(nums))
# Test case 3
nums = [4, 1, 2]
# Print the original list of numbers.
print("\nOriginal list of numbers:", nums)
# Print whether every even index contains an even number and every odd index contains an odd number.
print("Check whether every even index contains an even number and every \nodd index contains odd number of a given list:")
print(odd_even_position(nums))
Sample Output:
Original list of numbers: [2, 1, 4, 3, 6, 7, 6, 3] Check whether every even index contains an even number and every odd index contains odd number of a given list: True Original list of numbers: [2, 1, 4, 3, 6, 7, 6, 4] Check whether every even index contains an even number and every odd index contains odd number of a given list: False Original list of numbers: [2, 1, 4, 3, 6, 7, 6, 4] Check whether every even index contains an even number and every odd index contains odd number of a given list: True
Explanation:
Here is a breakdown of the above Python code:
- Function definition:
- The code defines a function named "odd_even_position()" that takes a list of numbers (nums) as an argument.
- all() Function and Generator Expression:
- The function uses the "all()" function along with a generator expression to check if every even index contains an even number and every odd index contains an odd number in the given list.
Visual Presentation:
Flowchart:
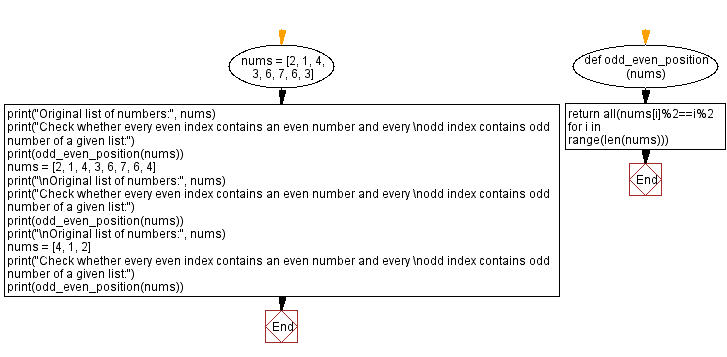
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to find the largest product of the pair of adjacent elements from a given list of integers.
Next: Write a Python program to check whether a given number is a narcissistic number or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics