Python: Test if the letters in the second string are present in the first string
Check Second String in First
Write a Python program that accepts two strings and determines whether the letters in the second string are present in the first string.
Sample Solution:
Python Code:
# Define a function named string_letter_check that takes two strings, str1 and str2, as arguments.
def string_letter_check(str1, str2):
# Check if all characters in str2 (ignoring case) are present in str1 (ignoring case).
return all([char in str1.lower() for char in str2.lower()])
# Test the function with different pairs of strings and print the results.
print(string_letter_check("python", "ypth")) # Expecting True (all characters in str2 are in str1)
print(string_letter_check("python", "ypths")) # Expecting False (not all characters in str2 are in str1)
print(string_letter_check("python", "ypthon")) # Expecting True (all characters in str2 are in str1)
print(string_letter_check("123456", "01234")) # Expecting False (not all characters in str2 are in str1)
print(string_letter_check("123456", "1234")) # Expecting True (all characters in str2 are in str1)
Sample Output:
True False True False True
Explanation:
Here is a breakdown of the above Python code:
- Function definition:
- The code defines a function named "string_letter_check()" that takes two strings, 'str1' and 'str2', as arguments.
- Return Statement:
- The function returns 'True' if all characters in 'str2' (ignoring case) are present in 'str1' (ignoring case). Otherwise, it returns 'False'.
- Test cases:
- The function is tested with different pairs of strings using print(string_letter_check(...))
Visual Presentation:
Flowchart:
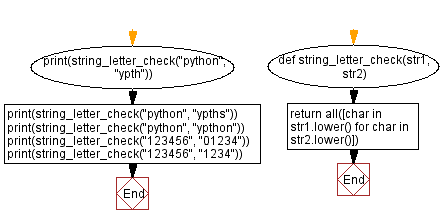
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to check whether a given employee code is exactly 8 digits or 12 digits.
Next: Write a Python program to compute the sum of the three lowest positive numbers from a given list of numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics