Python: Print a given N by M matrix of numbers line by line in forward > backwards > forward >... order
Python Basic - 1: Exercise-78 with Solution
Write a Python program to print a given N by M matrix of numbers line by line in forward > backwards > forward >... order.
Input matrix:
[[1, 2, 3, 4],
[5, 6, 7, 8],
[0, 6, 2, 8],
[2, 3, 0, 2]]
Output:
1
2
3
4
8
7
6
5
0
6
2
8
2
0
3
2
Sample Solution:
Python Code:
# Define a function to print a matrix in a zigzag pattern
def print_matrix(nums):
# Initialize a flag to determine the printing direction
flag = True
# Iterate through each line in the matrix
for line in nums:
# Check the flag to determine the printing direction
if flag == True:
# If flag is True, print the elements from left to right
i = 0
while i < len(line):
print(line[i])
i += 1
# Update the flag for the next line
flag = False
else:
# If flag is False, print the elements from right to left
i = -1
while i > -1 * len(line) - 1:
print(line[i])
i = i - 1
# Update the flag for the next line
flag = True
# Test the function with a matrix and print the elements in a zigzag pattern
print_matrix([[1, 2, 3, 4],
[5, 6, 7, 8],
[0, 6, 2, 8],
[2, 3, 0, 2]])
Sample Output:
1 2 3 4 8 7 6 5 0 6 2 8 2 0 3 2
Explanation:
Here is a breakdown of the above Python code:
- The function "print_matrix()" prints the elements of a matrix in a zigzag pattern.
- It initializes a flag ('flag') to determine the printing direction.
- It iterates through each line in the matrix ('nums').
- Depending on the flag, it either prints the elements from left to right or from right to left.
- After printing a line, it updates the flag for the next line.
- The function is tested with a sample matrix, and the elements are printed in a zigzag pattern.
Flowchart:
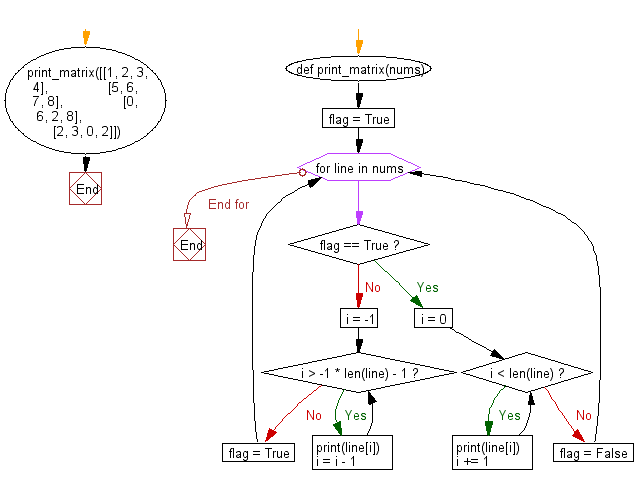
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to find the maximum profit in one transaction.
Next: Write a Python program to compute the largest product of three integers from a given list of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/basic/python-basic-1-exercise-78.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics